
Picture by Writer
A Convolutional Neural Community (CNN or ConvNet) is a deep studying algorithm particularly designed for duties the place object recognition is essential – like picture classification, detection, and segmentation. CNNs are in a position to obtain state-of-the-art accuracy on advanced imaginative and prescient duties, powering many real-life purposes comparable to surveillance techniques, warehouse administration, and extra.
As people, we are able to simply acknowledge objects in photos by analyzing patterns, shapes, and colours. CNNs might be educated to carry out this recognition too, by studying which patterns are necessary for differentiation. For instance, when attempting to differentiate between a photograph of a Cat versus a Canine, our mind focuses on distinctive form, textures, and facial options. A CNN learns to select up on these similar varieties of distinguishing traits. Even for very fine-grained categorization duties, CNNs are in a position to be taught advanced characteristic representations straight from pixels.
On this weblog publish, we’ll study Convolutional Neural Networks and the right way to use them to construct a picture classifier with PyTorch.
Convolutional neural networks (CNNs) are generally used for picture classification duties. At a excessive degree, CNNs include three predominant varieties of layers:
- Convolutional layers. Apply convolutional filters to the enter to extract options. The neurons in these layers are known as filters and seize spatial patterns within the enter.
- Pooling layers. Downsample the characteristic maps from the convolutional layers to consolidate info. Max pooling and common pooling are generally used methods.
- Totally-connected layers. Take the high-level options from the convolutional and pooling layers as enter for classification. A number of fully-connected layers might be stacked.
The convolutional filters act as characteristic detectors, studying to activate once they see particular varieties of patterns or shapes within the enter picture. As these filters are utilized throughout the picture, they produce characteristic maps that spotlight the place sure options are current.
For instance, one filter may activate when it sees vertical traces, producing a characteristic map displaying the vertical traces within the picture. A number of filters utilized to the identical enter produce a stack of characteristic maps, capturing totally different facets of the picture.
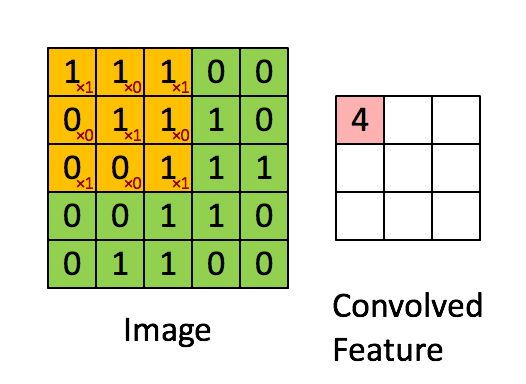
Gif by IceCream Labs
By stacking a number of convolutional layers, a CNN can be taught hierarchies of options – build up from easy edges and patterns to extra advanced shapes and objects. The pooling layers assist consolidate the characteristic representations and supply translational invariance.
The ultimate fully-connected layers take these discovered characteristic representations and use them for classification. For a picture classification job, the output layer sometimes makes use of a softmax activation to supply a chance distribution over lessons.
In PyTorch, we are able to outline the convolutional, pooling, and fully-connected layers to construct up a CNN structure. Right here is a few pattern code:
# Conv layers
self.conv1 = nn.Conv2d(in_channels, out_channels, kernel_size)
self.conv2 = nn.Conv2d(in_channels, out_channels, kernel_size)
# Pooling layer
self.pool = nn.MaxPool2d(kernel_size)
# Totally-connected layers
self.fc1 = nn.Linear(in_features, out_features)
self.fc2 = nn.Linear(in_features, out_features)
We are able to then practice the CNN on picture information, utilizing backpropagation and optimization. The convolutional and pooling layers will routinely be taught efficient characteristic representations, permitting the community to attain sturdy efficiency on imaginative and prescient duties.
On this part, we’ll load CIFAR10 and construct and practice a CNN-based classification mannequin utilizing PyTorch. The CIFAR10 dataset offers 32×32 RGB photos throughout ten lessons, which is helpful for testing picture classification fashions. There are ten lessons labeled in integers 0 to 9.
Observe: The instance code is the modified model from MachineLearningMastery.com weblog.
First, we’ll use torchvision to obtain and cargo the CIFAR10 dataset. We can even use torchvision to remodel each the testing and coaching units to tensors.
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision
remodel = torchvision.transforms.Compose(
[torchvision.transforms.ToTensor()]
)
practice = torchvision.datasets.CIFAR10(
root="information", practice=True, obtain=True, remodel=remodel
)
check = torchvision.datasets.CIFAR10(
root="information", practice=False, obtain=True, remodel=remodel
)
Downloading https://www.cs.toronto.edu/~kriz/cifar-10-python.tar.gz to information/cifar-10-python.tar.gz
100%|██████████| 170498071/170498071 [00:10<00:00, 15853600.54it/s]
Extracting information/cifar-10-python.tar.gz to information
Information already downloaded and verified
After that, we’ll use a knowledge loader and break up the photographs into the batches.
batch_size = 32
trainloader = torch.utils.information.DataLoader(
practice, batch_size=batch_size, shuffle=True
)
testloader = torch.utils.information.DataLoader(
check, batch_size=batch_size, shuffle=True
)
To visualise the picture in a single batch of the photographs, we’ll use matplotlib and torchvision utility perform.
from torchvision.utils import make_grid
import matplotlib.pyplot as plt
def show_batch(dl):
for photos, labels in dl:
fig, ax = plt.subplots(figsize=(12, 12))
ax.set_xticks([]); ax.set_yticks([])
ax.imshow(make_grid(photos[:64], nrow=8).permute(1, 2, 0))
break
show_batch(trainloader)
As we are able to see, we now have photos of automobiles, animals, planes, and boats.
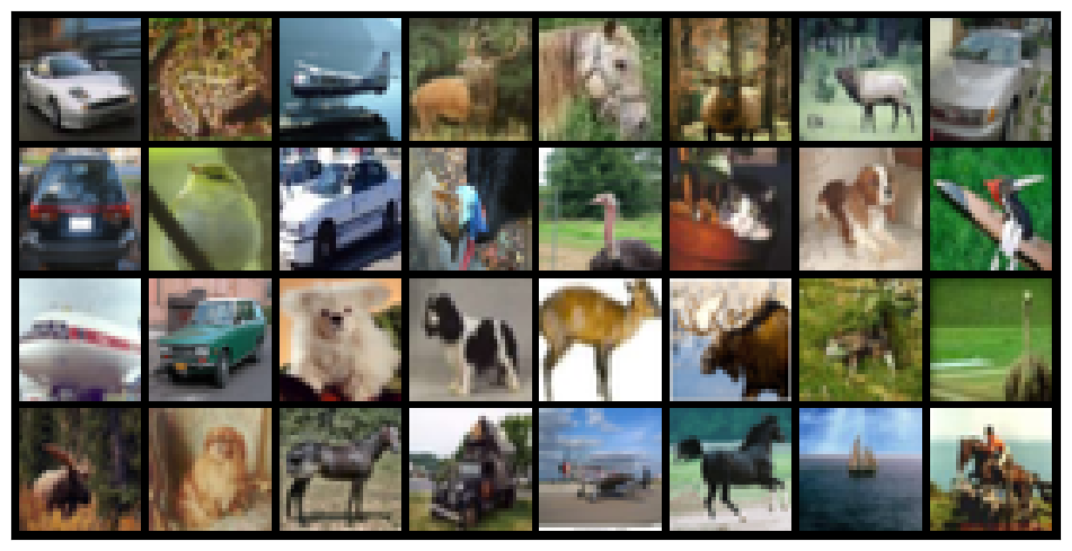
Subsequent, we’ll construct our CNN mannequin. For that, we now have to create a Python class and initialize the convolutions, maxpool, and totally linked layers. Our structure has 2 convolutional layers with pooling and linear layers.
After initializing, we is not going to join all of the layers sequentially within the ahead perform. In case you are new to PyTorch, it’s best to learn Interpretable Neural Networks with PyTorch to know every element intimately.
class CNNModel(nn.Module):
def __init__(self):
tremendous().__init__()
self.conv1 = nn.Conv2d(3, 32, kernel_size=(3,3), stride=1, padding=1)
self.act1 = nn.ReLU()
self.drop1 = nn.Dropout(0.3)
self.conv2 = nn.Conv2d(32, 32, kernel_size=(3,3), stride=1, padding=1)
self.act2 = nn.ReLU()
self.pool2 = nn.MaxPool2d(kernel_size=(2, 2))
self.flat = nn.Flatten()
self.fc3 = nn.Linear(8192, 512)
self.act3 = nn.ReLU()
self.drop3 = nn.Dropout(0.5)
self.fc4 = nn.Linear(512, 10)
def ahead(self, x):
# enter 3x32x32, output 32x32x32
x = self.act1(self.conv1(x))
x = self.drop1(x)
# enter 32x32x32, output 32x32x32
x = self.act2(self.conv2(x))
# enter 32x32x32, output 32x16x16
x = self.pool2(x)
# enter 32x16x16, output 8192
x = self.flat(x)
# enter 8192, output 512
x = self.act3(self.fc3(x))
x = self.drop3(x)
# enter 512, output 10
x = self.fc4(x)
return x
We are going to now initialize our mannequin, set loss perform, and optimizer.
mannequin = CNNModel()
loss_fn = nn.CrossEntropyLoss()
optimizer = optim.SGD(mannequin.parameters(), lr=0.001, momentum=0.9)
Within the coaching section, we’ll practice our mannequin for 10 epochs.
- We’re utilizing the ahead perform of the mannequin for a ahead go, then a backward go utilizing the loss perform, and eventually updating the weights. This step is sort of related in every kind of neural community fashions.
- After that, we’re utilizing a check information loader to guage mannequin efficiency on the finish of every epoch.
- Calculating the accuracy of the mannequin and printing the outcomes.
n_epochs = 10
for epoch in vary(n_epochs):
for i, (photos, labels) in enumerate(trainloader):
# Ahead go
outputs = mannequin(photos)
loss = loss_fn(outputs, labels)
# Backward go and optimize
optimizer.zero_grad()
loss.backward()
optimizer.step()
right = 0
whole = 0
with torch.no_grad():
for photos, labels in testloader:
outputs = mannequin(photos)
_, predicted = torch.max(outputs.information, 1)
whole += labels.measurement(0)
right += (predicted == labels).sum().merchandise()
print('Epoch %d: Accuracy: %d %%' % (epoch,(100 * right / whole)))
Our easy mannequin has achieved 57% accuracy, which is unhealthy. However, you may enhance the mannequin efficiency by including extra layers, working it for extra epochs, and hyperparameter optimization.
Epoch 0: Accuracy: 41 %
Epoch 1: Accuracy: 46 %
Epoch 2: Accuracy: 48 %
Epoch 3: Accuracy: 50 %
Epoch 4: Accuracy: 52 %
Epoch 5: Accuracy: 53 %
Epoch 6: Accuracy: 53 %
Epoch 7: Accuracy: 56 %
Epoch 8: Accuracy: 56 %
Epoch 9: Accuracy: 57 %
With PyTorch, you do not have to create all of the parts of convolutional neural networks from scratch as they’re already out there. It turns into even less complicated should you use `torch.nn.Sequential`. PyTorch is designed to be modular and presents better flexibility in constructing, coaching, and assessing neural networks.
On this publish, we explored the right way to construct and practice a convolutional neural community for picture classification utilizing PyTorch. We coated the core parts of CNN architectures – convolutional layers for characteristic extraction, pooling layers for downsampling, and fully-connected layers for prediction.
I hope this publish offered a useful overview of implementing convolutional neural networks with PyTorch. CNNs are elementary structure in deep studying for pc imaginative and prescient, and PyTorch provides us the pliability to rapidly construct, practice, and consider these fashions.
Abid Ali Awan (@1abidaliawan) is a licensed information scientist skilled who loves constructing machine studying fashions. At present, he’s specializing in content material creation and writing technical blogs on machine studying and information science applied sciences. Abid holds a Grasp’s diploma in Expertise Administration and a bachelor’s diploma in Telecommunication Engineering. His imaginative and prescient is to construct an AI product utilizing a graph neural community for college kids scuffling with psychological sickness.