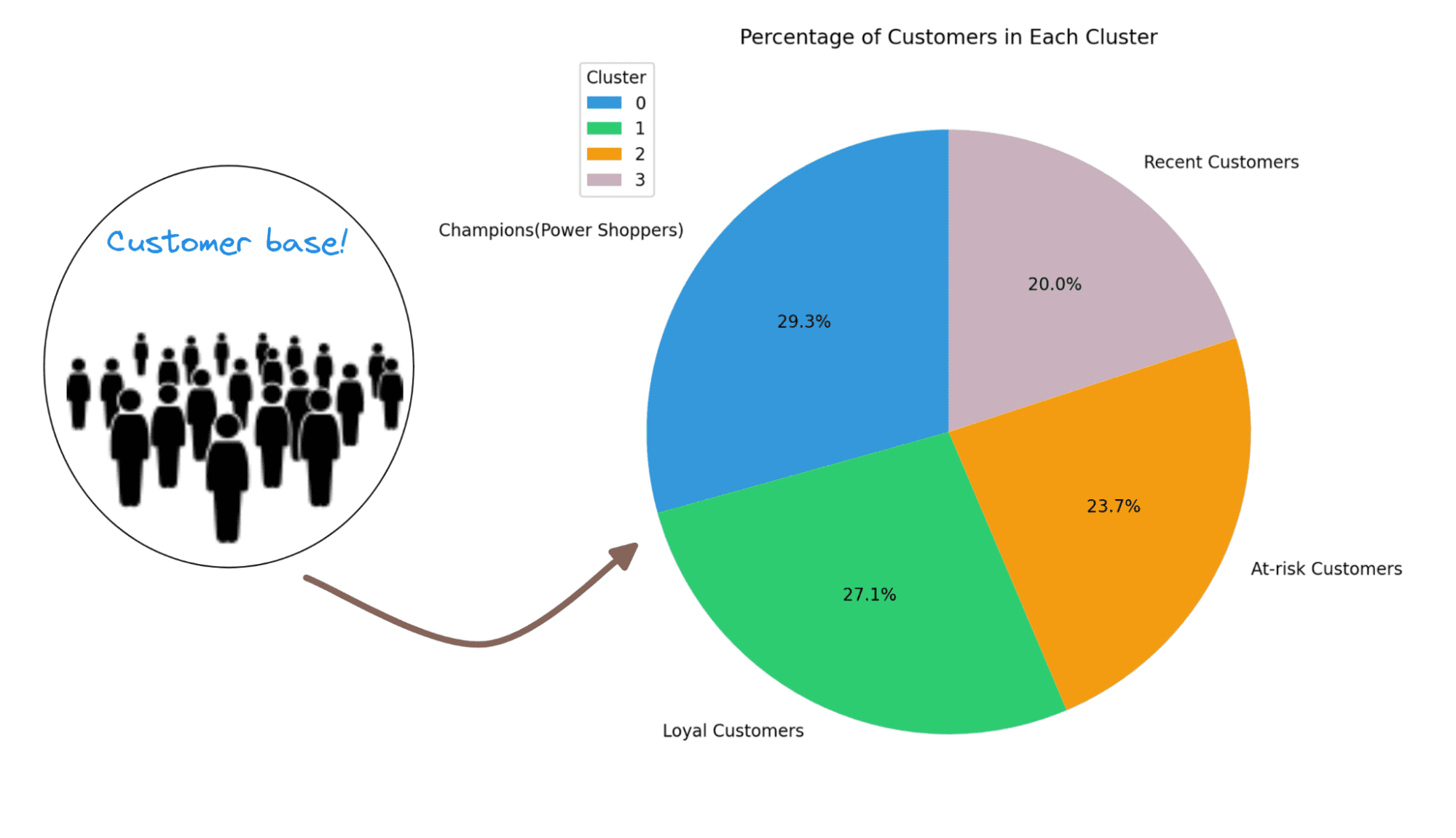
Picture by Writer | Created Utilizing Excalidraw and Flaticon
Buyer segmentation can assist companies tailor their advertising and marketing efforts and enhance buyer satisfaction. Right here’s how.
Functionally, buyer segmentation entails dividing a buyer base into distinct teams or segments—based mostly on shared traits and behaviors. By understanding the wants and preferences of every section, companies can ship extra personalised and efficient advertising and marketing campaigns, resulting in elevated buyer retention and income.
On this tutorial, we’ll discover buyer segmentation in Python by combining two elementary strategies: RFM (Recency, Frequency, Financial) evaluation and Okay-Means clustering. RFM evaluation offers a structured framework for evaluating buyer conduct, whereas Okay-means clustering gives a data-driven method to group clients into significant segments. We’ll work with a real-world dataset from the retail trade: the On-line Retail dataset from UCI machine studying repository.
From information preprocessing to cluster evaluation and visualization, we’ll code our manner by every step. So let’s dive in!
Let’s begin by stating our purpose: By making use of RFM evaluation and Okay-means clustering to this dataset, we’d like to achieve insights into buyer conduct and preferences.
RFM Evaluation is an easy but highly effective technique to quantify buyer conduct. It evaluates clients based mostly on three key dimensions:
- Recency (R): How just lately did a selected buyer make a purchase order?
- Frequency (F): How typically do they make purchases?
- Financial Worth (M): How a lot cash do they spend?
We’ll use the knowledge within the dataset to compute the recency, frequency, and financial values. Then, we’ll map these values to the commonly used RFM rating scale of 1 – 5.
If you happen to’d like, you possibly can discover and analyze additional utilizing these RFM scores. However we’ll attempt to establish buyer segments with related RFM traits. And for this, we’ll use Okay-Means clustering, an unsupervised machine studying algorithm that teams related information factors into clusters.
So let’s begin coding!
🔗 Hyperlink to Google Colab pocket book.
Step 1 – Import Mandatory Libraries and Modules
First, let’s import the mandatory libraries and the particular modules as wanted:
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.cluster import KMeans
We’d like pandas and matplotlib for information exploration and visualization, and the KMeans
class from scikit-learn’s cluster module to carry out Okay-Means clustering.
Step 2 – Load the Dataset
As talked about, we’ll use the On-line Retail dataset. The dataset comprises buyer information: transactional data, together with buy dates, portions, costs, and buyer IDs.
Let’s learn within the information that’s initially in an excel file from its URL right into a pandas dataframe.
# Load the dataset from UCI repository
url = "https://archive.ics.uci.edu/ml/machine-learning-databases/00352/Onlinepercent20Retail.xlsx"
information = pd.read_excel(url)
Alternatively, you possibly can obtain the dataset and skim the excel file right into a pandas dataframe.
Step 3 – Discover and Clear the Dataset
Now let’s begin exploring the dataset. Have a look at the primary few rows of the dataset:
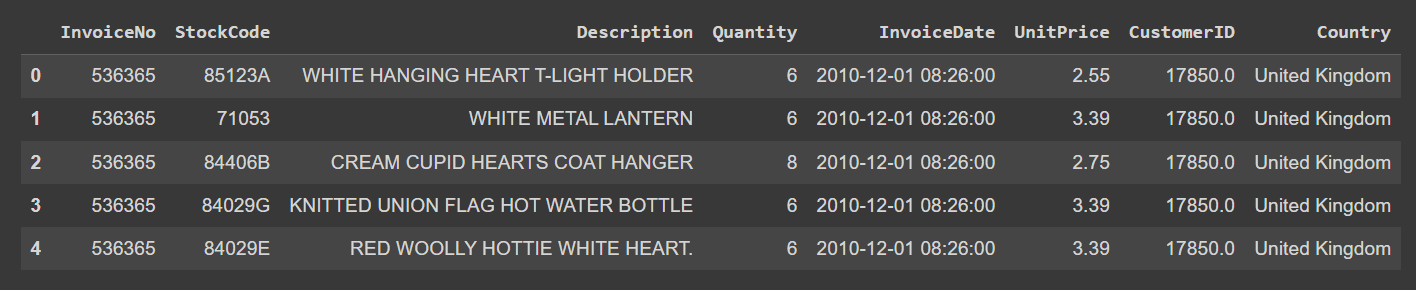
Output of information.head()
Now name the describe()
technique on the dataframe to grasp the numerical options higher:
We see that the “CustomerID” column is at the moment a floating level worth. Once we clear the info, we’ll forged it into an integer:
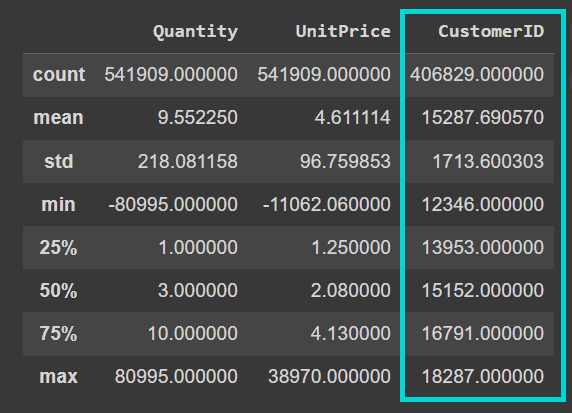
Output of information.describe()
Additionally be aware that the dataset is kind of noisy. The “Amount” and “UnitPrice” columns include adverse values:
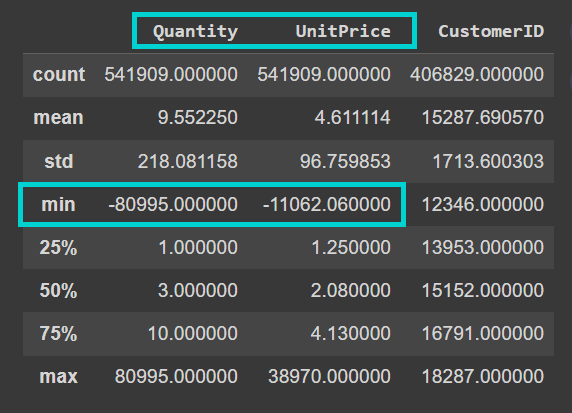
Output of information.describe()
Let’s take a more in-depth take a look at the columns and their information varieties:
We see that the dataset has over 541K information and the “Description” and “CustomerID” columns include lacking values:
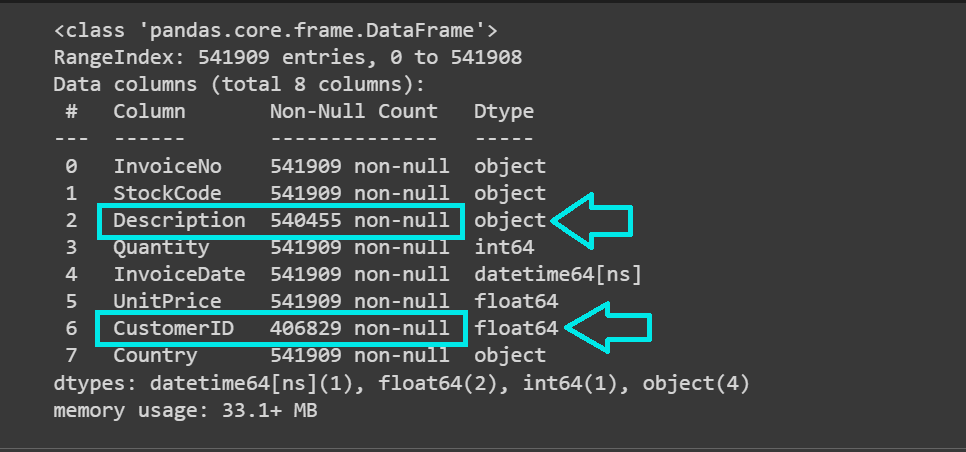
Let’s get the rely of lacking values in every column:
# Verify for lacking values in every column
missing_values = information.isnull().sum()
print(missing_values)
As anticipated, the “CustomerID” and “Description” columns include lacking values:
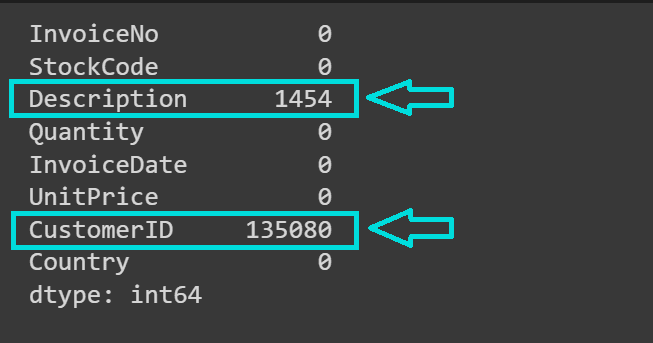
For our evaluation, we don’t want the product description contained within the “Description” column. Nevertheless, we’d like the “CustomerID” for the following steps in our evaluation. So let’s drop the information with lacking “CustomerID”:
# Drop rows with lacking CustomerID
information.dropna(subset=['CustomerID'], inplace=True)
Additionally recall that the values “Amount” and “UnitPrice” columns must be strictly non-negative. However they include adverse values. So let’s additionally drop the information with adverse values for “Amount” and “UnitPrice”:
# Take away rows with adverse Amount and Worth
information = information[(data['Quantity'] > 0) & (information['UnitPrice'] > 0)]
Let’s additionally convert the “CustomerID” to an integer:
information['CustomerID'] = information['CustomerID'].astype(int)
# Confirm the info sort conversion
print(information.dtypes)
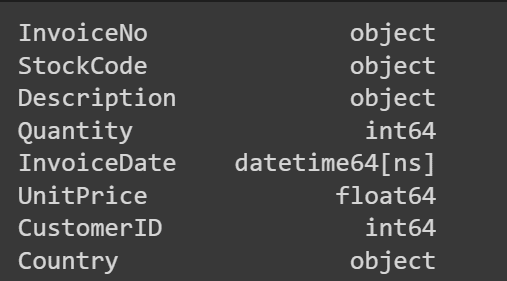
Step 4 – Compute Recency, Frequency, and Financial Worth
Let’s begin out by defining a reference date snapshot_date
that’s a day later than the latest date within the “InvoiceDate” column:
snapshot_date = max(information['InvoiceDate']) + pd.DateOffset(days=1)
Subsequent, create a “Complete” column that comprises Amount*UnitPrice for all of the information:
information['Total'] = information['Quantity'] * information['UnitPrice']
To calculate the Recency, Frequency, and MonetaryValue, we calculate the next—grouped by CustomerID:
- For recency, we’ll calculate the distinction between the latest buy date and a reference date (
snapshot_date
). This provides the variety of days for the reason that buyer’s final buy. So smaller values point out {that a} buyer has made a purchase order extra just lately. However once we speak about recency scores, we’d need clients who purchased just lately to have the next recency rating, sure? We’ll deal with this within the subsequent step. - As a result of frequency measures how typically a buyer makes purchases, we’ll calculate it as the entire variety of distinctive invoices or transactions made by every buyer.
- Financial worth quantifies how a lot cash a buyer spends. So we’ll discover the common of the entire financial worth throughout transactions.
rfm = information.groupby('CustomerID').agg({
'InvoiceDate': lambda x: (snapshot_date - x.max()).days,
'InvoiceNo': 'nunique',
'Complete': 'sum'
})
Let’s rename the columns for readability:
rfm.rename(columns={'InvoiceDate': 'Recency', 'InvoiceNo': 'Frequency', 'Complete': 'MonetaryValue'}, inplace=True)
rfm.head()
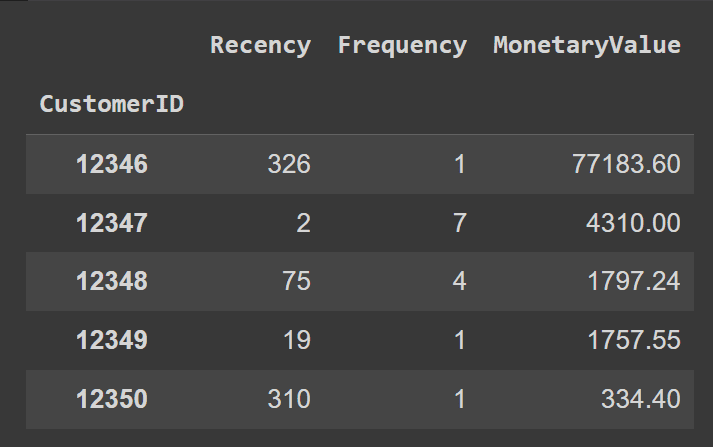
Step 5 – Map RFM Values onto a 1-5 Scale
Now let’s map the “Recency”, “Frequency”, and “MonetaryValue” columns to tackle values in a scale of 1-5; one in every of {1,2,3,4,5}.
We’ll basically assign the values to 5 totally different bins, and map every bin to a worth. To assist us repair the bin edges, let’s use the quantile values of the “Recency”, “Frequency”, and “MonetaryValue” columns:
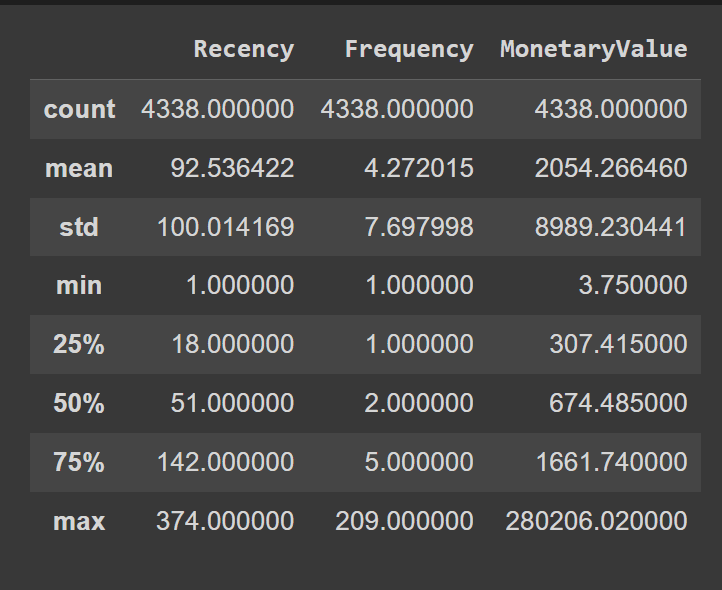
Right here’s how we outline the customized bin edges:
# Calculate customized bin edges for Recency, Frequency, and Financial scores
recency_bins = [rfm['Recency'].min()-1, 20, 50, 150, 250, rfm['Recency'].max()]
frequency_bins = [rfm['Frequency'].min() - 1, 2, 3, 10, 100, rfm['Frequency'].max()]
monetary_bins = [rfm['MonetaryValue'].min() - 3, 300, 600, 2000, 5000, rfm['MonetaryValue'].max()]
Now that we’ve outlined the bin edges, let’s map the scores to corresponding labels between 1 and 5 (each inclusive):
# Calculate Recency rating based mostly on customized bins
rfm['R_Score'] = pd.lower(rfm['Recency'], bins=recency_bins, labels=vary(1, 6), include_lowest=True)
# Reverse the Recency scores in order that greater values point out newer purchases
rfm['R_Score'] = 5 - rfm['R_Score'].astype(int) + 1
# Calculate Frequency and Financial scores based mostly on customized bins
rfm['F_Score'] = pd.lower(rfm['Frequency'], bins=frequency_bins, labels=vary(1, 6), include_lowest=True).astype(int)
rfm['M_Score'] = pd.lower(rfm['MonetaryValue'], bins=monetary_bins, labels=vary(1, 6), include_lowest=True).astype(int)
Discover that the R_Score, based mostly on the bins, is 1 for latest purchases 5 for all purchases remodeled 250 days in the past. However we’d like the latest purchases to have an R_Score of 5 and purchases remodeled 250 days in the past to have an R_Score of 1.
To realize the specified mapping, we do: 5 - rfm['R_Score'].astype(int) + 1
.
Let’s take a look at the primary few rows of the R_Score, F_Score, and M_Score columns:
# Print the primary few rows of the RFM DataFrame to confirm the scores
print(rfm[['R_Score', 'F_Score', 'M_Score']].head(10))
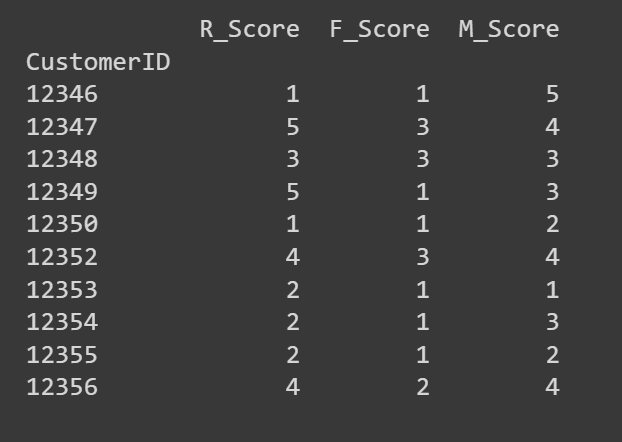
If you happen to’d like, you need to use these R, F, and M scores to hold out an in-depth evaluation. Or use clustering to establish segments with related RFM traits. We’ll select the latter!
Step 6 – Carry out Okay-Means Clustering
Okay-Means clustering is delicate to the size of options. As a result of the R, F, and M values are all on the identical scale, we are able to proceed to carry out clustering with out additional scaling the options.
Let’s extract the R, F, and M scores to carry out Okay-Means clustering:
# Extract RFM scores for Okay-means clustering
X = rfm[['R_Score', 'F_Score', 'M_Score']]
Subsequent, we have to discover the optimum variety of clusters. For this let’s run the Okay-Means algorithm for a spread of Okay values and use the elbow technique to select the optimum Okay:
# Calculate inertia (sum of squared distances) for various values of ok
inertia = []
for ok in vary(2, 11):
kmeans = KMeans(n_clusters=ok, n_init= 10, random_state=42)
kmeans.match(X)
inertia.append(kmeans.inertia_)
# Plot the elbow curve
plt.determine(figsize=(8, 6),dpi=150)
plt.plot(vary(2, 11), inertia, marker="o")
plt.xlabel('Variety of Clusters (ok)')
plt.ylabel('Inertia')
plt.title('Elbow Curve for Okay-means Clustering')
plt.grid(True)
plt.present()
We see that the curve elbows out at 4 clusters. So let’s divide the shopper base into 4 segments.

We’ve fastened Okay to 4. So let’s run the Okay-Means algorithm to get the cluster assignments for all factors within the dataset:
# Carry out Okay-means clustering with greatest Okay
best_kmeans = KMeans(n_clusters=4, n_init=10, random_state=42)
rfm['Cluster'] = best_kmeans.fit_predict(X)
Step 7 – Interpret the Clusters to Establish Buyer Segments
Now that we’ve got the clusters, let’s attempt to characterize them based mostly on the RFM scores.
# Group by cluster and calculate imply values
cluster_summary = rfm.groupby('Cluster').agg({
'R_Score': 'imply',
'F_Score': 'imply',
'M_Score': 'imply'
}).reset_index()
The common R, F, and M scores for every cluster ought to already offer you an thought of the traits.
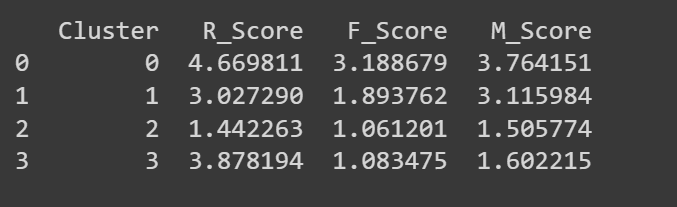
However let’s visualize the common R, F, and M scores for the clusters so it’s simple to interpret:
colours = ['#3498db', '#2ecc71', '#f39c12','#C9B1BD']
# Plot the common RFM scores for every cluster
plt.determine(figsize=(10, 8),dpi=150)
# Plot Avg Recency
plt.subplot(3, 1, 1)
bars = plt.bar(cluster_summary.index, cluster_summary['R_Score'], shade=colours)
plt.xlabel('Cluster')
plt.ylabel('Avg Recency')
plt.title('Common Recency for Every Cluster')
plt.grid(True, linestyle="--", alpha=0.5)
plt.legend(bars, cluster_summary.index, title="Clusters")
# Plot Avg Frequency
plt.subplot(3, 1, 2)
bars = plt.bar(cluster_summary.index, cluster_summary['F_Score'], shade=colours)
plt.xlabel('Cluster')
plt.ylabel('Avg Frequency')
plt.title('Common Frequency for Every Cluster')
plt.grid(True, linestyle="--", alpha=0.5)
plt.legend(bars, cluster_summary.index, title="Clusters")
# Plot Avg Financial
plt.subplot(3, 1, 3)
bars = plt.bar(cluster_summary.index, cluster_summary['M_Score'], shade=colours)
plt.xlabel('Cluster')
plt.ylabel('Avg Financial')
plt.title('Common Financial Worth for Every Cluster')
plt.grid(True, linestyle="--", alpha=0.5)
plt.legend(bars, cluster_summary.index, title="Clusters")
plt.tight_layout()
plt.present()
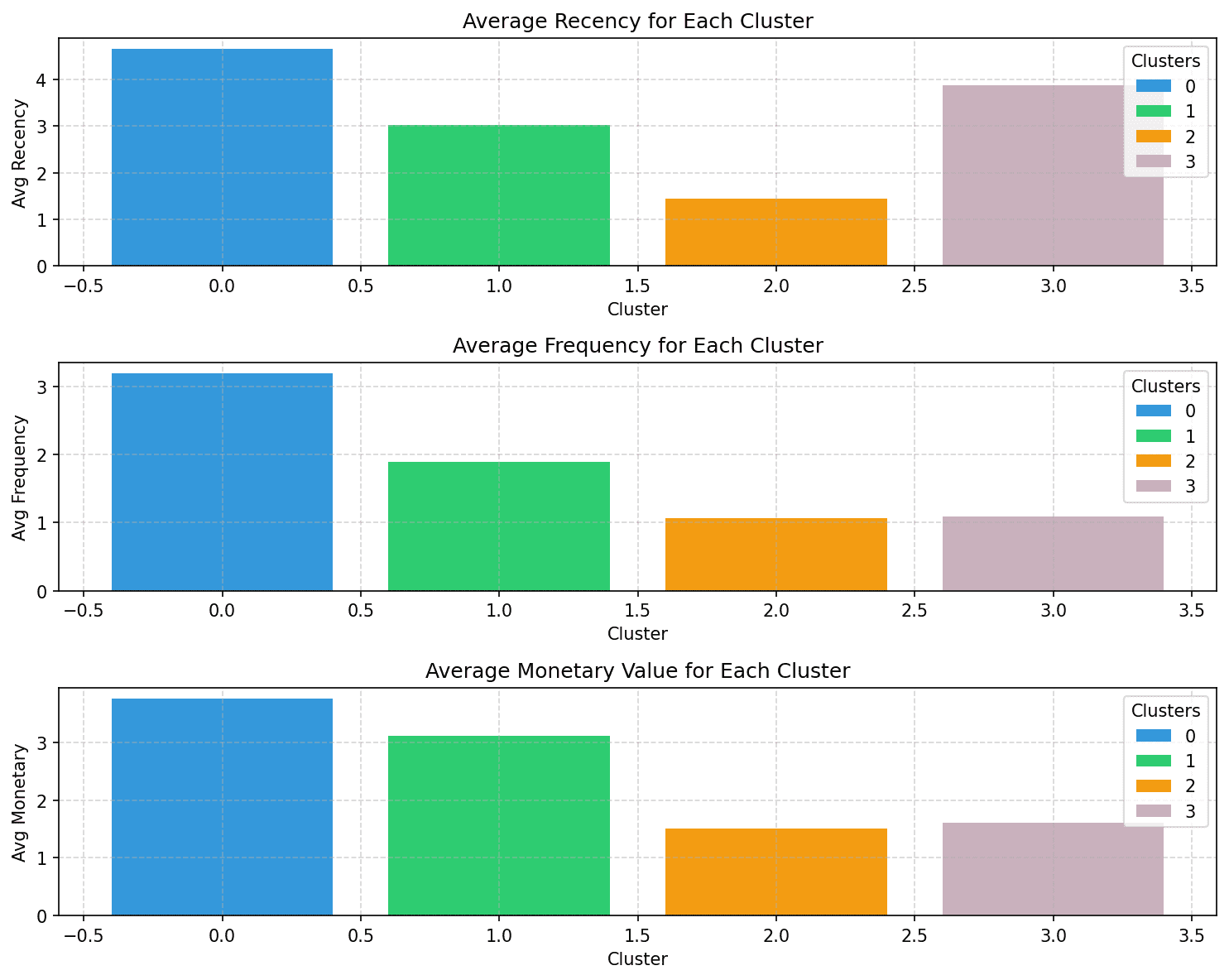
Discover how the shoppers in every of the segments might be characterised based mostly on the recency, frequency, and financial values:
- Cluster 0: Of all of the 4 clusters, this cluster has the highest recency, frequency, and financial values. Let’s name the shoppers on this cluster champions (or energy buyers).
- Cluster 1: This cluster is characterised by average recency, frequency, and financial values. These clients nonetheless spend extra and buy extra continuously than clusters 2 and three. Let’s name them loyal clients.
- Cluster 2: Clients on this cluster are inclined to spend much less. They don’t purchase typically, and haven’t made a purchase order just lately both. These are possible inactive or at-risk clients.
- Cluster 3: This cluster is characterised by excessive recency and comparatively decrease frequency and average financial values. So these are latest clients who can doubtlessly turn into long-term clients.
Listed here are some examples of how one can tailor advertising and marketing efforts—to focus on clients in every section—to boost buyer engagement and retention:
- For Champions/Energy Customers: Provide personalised particular reductions, early entry, and different premium perks to make them really feel valued and appreciated.
- For Loyal Clients: Appreciation campaigns, referral bonuses, and rewards for loyalty.
- For At-Danger Clients: Re-engagement efforts that embrace operating reductions or promotions to encourage shopping for.
- For Current Clients: Focused campaigns educating them concerning the model and reductions on subsequent purchases.
It’s additionally useful to grasp what proportion of shoppers are within the totally different segments. It will additional assist streamline advertising and marketing efforts and develop what you are promoting.
Let’s visualize the distribution of the totally different clusters utilizing a pie chart:
cluster_counts = rfm['Cluster'].value_counts()
colours = ['#3498db', '#2ecc71', '#f39c12','#C9B1BD']
# Calculate the entire variety of clients
total_customers = cluster_counts.sum()
# Calculate the share of shoppers in every cluster
percentage_customers = (cluster_counts / total_customers) * 100
labels = ['Champions(Power Shoppers)','Loyal Customers','At-risk Customers','Recent Customers']
# Create a pie chart
plt.determine(figsize=(8, 8),dpi=200)
plt.pie(percentage_customers, labels=labels, autopct="%1.1f%%", startangle=90, colours=colours)
plt.title('Proportion of Clients in Every Cluster')
plt.legend(cluster_summary['Cluster'], title="Cluster", loc="higher left")
plt.present()
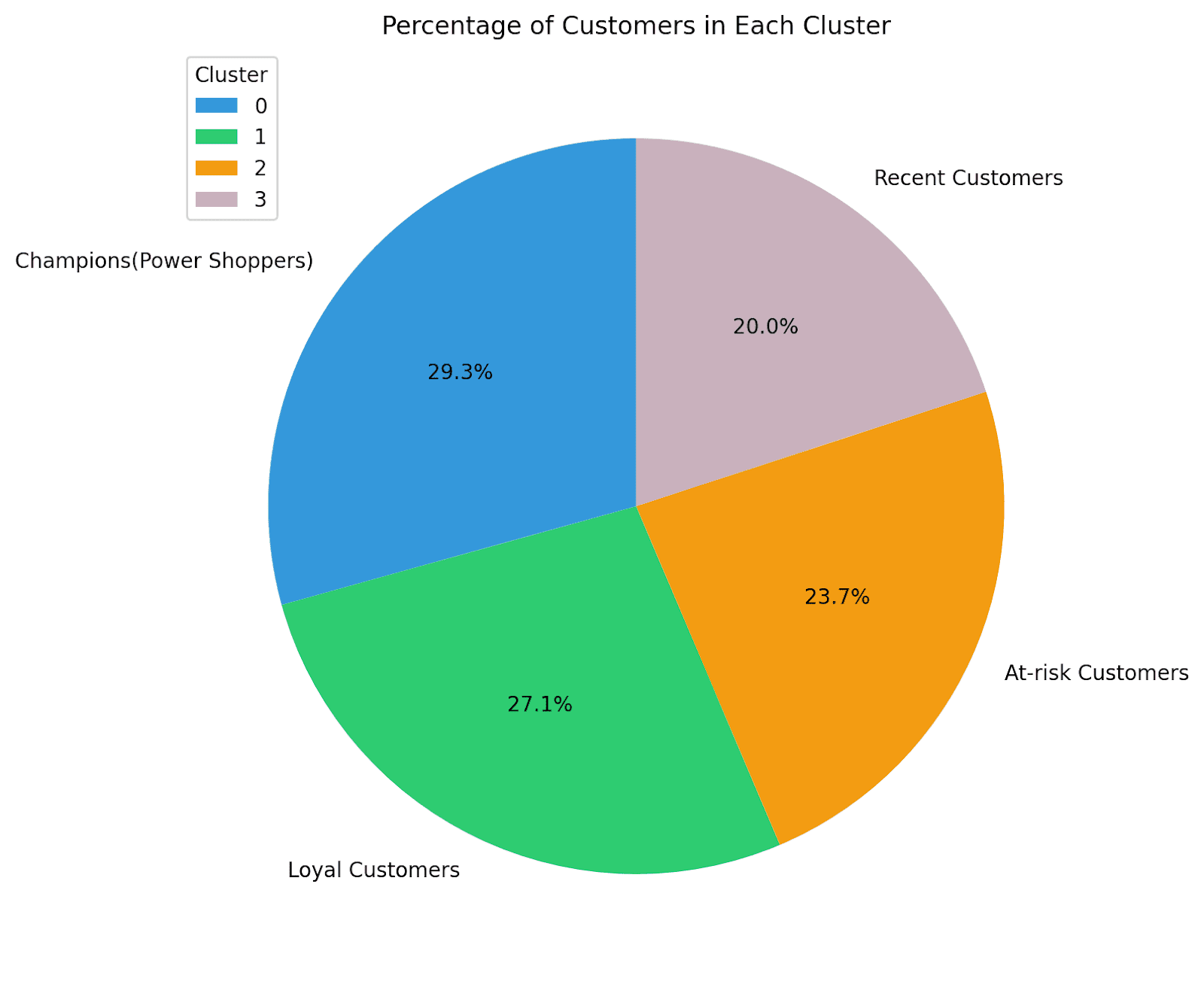
Right here we go! For this instance, we’ve got fairly a fair distribution of shoppers throughout segments. So we are able to make investments effort and time in retaining current clients, re-engaging with at-risk clients, and educating latest clients.
And that’s a wrap! We went from over 154K buyer information to 4 clusters in 7 simple steps. I hope you perceive how buyer segmentation permits you to make data-driven choices that affect enterprise progress and buyer satisfaction by permitting for:
- Personalization: Segmentation permits companies to tailor their advertising and marketing messages, product suggestions, and promotions to every buyer group’s particular wants and pursuits.
- Improved Concentrating on: By figuring out high-value and at-risk clients, companies can allocate sources extra effectively, focusing efforts the place they’re almost certainly to yield outcomes.
- Buyer Retention: Segmentation helps companies create retention methods by understanding what retains clients engaged and happy.
As a subsequent step, strive making use of this method to a different dataset, doc your journey, and share with the neighborhood! However bear in mind, efficient buyer segmentation and operating focused campaigns requires an excellent understanding of your buyer base—and the way the shopper base evolves. So it requires periodic evaluation to refine your methods over time.
The On-line Retail Dataset is licensed underneath a Artistic Commons Attribution 4.0 Worldwide (CC BY 4.0) license:
On-line Retail. (2015). UCI Machine Studying Repository. https://doi.org/10.24432/C5BW33.
Bala Priya C is a developer and technical author from India. She likes working on the intersection of math, programming, information science, and content material creation. Her areas of curiosity and experience embrace DevOps, information science, and pure language processing. She enjoys studying, writing, coding, and low! At present, she’s engaged on studying and sharing her data with the developer neighborhood by authoring tutorials, how-to guides, opinion items, and extra.
Bala Priya C is a developer and technical author from India. She likes working on the intersection of math, programming, information science, and content material creation. Her areas of curiosity and experience embrace DevOps, information science, and pure language processing. She enjoys studying, writing, coding, and low! At present, she’s engaged on studying and sharing her data with the developer neighborhood by authoring tutorials, how-to guides, opinion items, and extra.