An integral a part of ML Engineering is constructing dependable and scalable procedures for extracting knowledge, reworking it, enriching it and loading it in a particular file retailer or database. This is among the parts wherein the information scientist and the ML engineer collaborate essentially the most. Sometimes, the information scientist comes up with a tough model of what the information set ought to appear to be. Ideally, not on a Jupyter pocket book. Then, the ML engineer joins this job to assist making the code extra readable, environment friendly and dependable.
ML ETLs will be composed of a number of sub-ETLs or duties. And they are often materialized in very totally different kinds. Some frequent examples:
- Scala-based Spark job studying and processing occasion log knowledge saved in S3 as Parquet information and scheduled by means of Airflow on a weekly foundation.
- Python course of executing a Redshift SQL question by means of a scheduled AWS Lambda perform.
- Advanced pandas-heavy processing executed by means of a Sagemaker Processing Job utilizing EventBridge triggers.
We will establish totally different entities in these kind of ETLs, we’ve Sources (the place the uncooked knowledge lives), Locations (the place the ultimate knowledge artifact will get saved), Knowledge Processes (how the information will get learn, processed and loaded) and Triggers (how the ETLs get initiated).

- Below the Sources, we are able to have shops resembling AWS Redshift, AWS S3, Cassandra, Redis or exterior APIs. Locations are the identical.
- The Knowledge Processes are usually run underneath ephemeral Docker containers. We may add one other stage of abstraction utilizing Kubernetes or some other AWS managed service resembling AWS ECS or AWS Fargate. And even SageMaker Pipelines or Processing Jobs.You possibly can run these processes in a cluster by leveraging particular knowledge processing engines resembling Spark, Dask, Hive, Redshift SQL engine. Additionally, you should use easy single-instance processes utilizing Python processes and Pandas for knowledge processing. Other than that, there are another attention-grabbing frameworks resembling Polars, Vaex, Ray or Modin which will be helpful to sort out intermediate options.
- The preferred Set off instrument is Airflow. Others that can be utilized are Prefect, Dagster, Argo Workflows or Mage.

A framework is a set of abstractions, conventions and out-of-the-box utilities that can be utilized to create a extra uniform codebase when utilized to concrete issues. Frameworks are very handy for ETLs. As we’ve beforehand described, there are very generic entities that might probably be abstracted or encapsulated to generate complete workflows.
The development that I’d take to construct an inside knowledge processing framework is the next:
- Begin by constructing a library of connectors to the totally different Sources and Locations. Implement them as you want them all through the totally different tasks you’re employed on. That’s the easiest way to keep away from YAGNI.
- Create easy and automatic improvement workflow that lets you iterate rapidly the codebase. For instance, configure CI/CD workflows to mechanically check, lint and publish your package deal.
- Create utilities resembling studying SQL scripts, spinning up Spark periods, dates formatting capabilities, metadata turbines, logging utilities, capabilities for fetching credentials and connection parameters and alerting utilities amongst others.
- Select between constructing an inside framework for writing workflows or use an present one. The complexity scope is extensive when contemplating this in-house improvement. You can begin with some easy conventions when constructing workflows and find yourself constructing some DAG-based library with generic lessons resembling Luigi or Metaflow. These are standard frameworks that you should use.
This can be a crucial and central a part of your knowledge codebase. All of your processes will use this library to maneuver knowledge round from one supply into one other vacation spot. A strong and well-though preliminary software program design is vital.

However why would we need to do that? Effectively, the primary causes are:
- Reusability: Utilizing the identical software program parts in several software program tasks permits for larger productiveness. The piece of software program must be developed solely as soon as. Then, it may be built-in into different software program tasks. However this concept isn’t new. We will discover references again in 1968 on a convention whose purpose was to unravel the so-called software program disaster.
- Encapsulation: Not all of the internals of the totally different connectors used by means of the library have to be proven to end-users. Therefore, by offering an comprehensible interface, that’s sufficient. For instance, if we had a connector to a database, we wouldn’t like that the connection string obtained uncovered as a public attribute of the connector class. By utilizing a library we are able to be sure that safe entry to knowledge sources is assured. Evaluation this bit
- Increased-quality codebase: We have now to develop assessments solely as soon as. Therefore, builders can depend on the library as a result of it incorporates a check suite (Ideally, with a really excessive check protection). When debugging for errors or points we are able to ignore, at the very least at first move, that the difficulty is inside the library if we’re assured on our check suite.
- Standardisation / “Opinionation”: Having a library of connectors determines, in sure method, the way in which you develop ETLs. That’s good, as a result of ETLs within the group could have the identical methods of extracting or writing knowledge into the totally different knowledge sources. Standardisation results in higher communication, extra productiveness and higher forecasting and planning.
When constructing one of these library, groups commit to keep up it over time and assume the chance of getting to implement complicated refactors when wanted. Some causes of getting to do these refactors could be:
- The organisation migrates to a unique public cloud.
- The information warehouse engine adjustments.
- New dependency model breaks interfaces.
- Extra safety permission checks have to be put in place.
- A brand new crew is available in with totally different opinions in regards to the library design.a
Interface lessons
If you wish to make your ETLs agnostic of the Sources or Locations, it’s a good choice to create interface lessons for base entities. Interfaces function template definitions.
For instance, you’ll be able to have summary lessons for outlining required strategies and attributes of a DatabaseConnector. Let’s present a simplified instance of how this class may appear to be:
from abc import ABC
class DatabaseConnector(ABC):
def __init__(self, connection_string: str):
self.connection_string = connection_string
@abc.abstractmethod
def join(self):
move
@abc.abstractmethod
def execute(self, sql: str):
move
Different builders would subclass from the DatabaseConnector and create new concrete implementations. As an illustration, a MySqlConnector or CassandraDbConnector may very well be carried out on this vogue. This may assist end-users to rapidly perceive use any connector subclassed from the DatabaseConnector as all of them could have the identical interface (identical strategies).
mysql = MySqlConnector(connection_string)
mysql.join()
mysql.execute("SELECT * FROM public.desk")
cassandra = CassandraDbConnector(connection_string)
cassandra.join()
cassandra.execute("SELECT * FROM public.desk")
Simples interfaces with well-named strategies are very highly effective and permit for higher productiveness. So my recommendation is to spend high quality time desirous about it.
The proper documentation
Documentation not solely refers to docstrings and inline feedback within the code. It additionally refers back to the surrounding explanations you give in regards to the library. Writing a daring assertion about what’s the tip purpose of the package deal and a pointy clarification of the necessities and pointers to contribute is crucial.
For instance:
"This utils library will likely be used throughout all of the ML knowledge pipelines and have engineering jobs to supply easy and dependable connectors to the totally different methods within the group".
Or
"This library incorporates a set of characteristic engineering strategies, transformations and algorithms that can be utilized out-of-the-box with a easy interface that may be chained in a scikit-learn-type of pipeline".
Having a transparent mission of the library paves the way in which for an accurate interpretation from contributors. That is why open supply libraries (E.g: pandas, scikit-learn, and so on) have gained such an important recognition these final years. Contributors have embraced the purpose of the library and they’re dedicated to comply with the outlined requirements. We ought to be doing one thing fairly comparable at organizations.
Proper after the mission is said, we must always develop the foundational software program structure. How do we wish our interfaces to appear to be? Ought to we cowl performance by means of extra flexibility within the interface strategies (e.g: extra arguments that result in totally different behaviours) or extra granular strategies (e.g: every technique has a really particular perform)?
After having that, the styleguide. Define the popular modules hierarchy, the documentation depth required, publish PRs, protection necessities, and so on.
With respect to documentation within the code, docstrings have to be sufficiently descriptive of the perform behaviour however we shouldn’t fall into simply copying the perform identify. Generally, the perform identify is sufficiently expressive {that a} docstring explaining its behaviour is simply redundant. Be concise and correct. Let’s present a dumb instance:
❌No!
class NeptuneDbConnector:
...
def shut():
"""This perform checks if the connection to the database
is opened. Whether it is, it closes it and if it doesn’t,
it does nothing.
"""
✅Sure!
class NeptuneDbConnector:
...
def shut():
"""Closes connection to the database."""
Coming to the subject of inline feedback, I all the time like to make use of them to clarify sure issues which may appear bizarre or irregular. Additionally, if I’ve to make use of a fancy logic or fancy syntax, it’s all the time higher for those who write a transparent clarification on high of that snippet.
# Getting the utmost integer of the checklist
l = [23, 49, 6, 32]
cut back((lambda x, y: x if x > y else y), l)
Other than that, you can even embody hyperlinks to Github points or Stackoverflow solutions. That is actually helpful, specifically for those who needed to code a bizarre logic simply to beat a recognized dependency concern. It’s also actually handy if you needed to implement an optimisation trick that you simply obtained from Stackoverflow.
These two, interface lessons and clear documentation are, for my part, the most effective methods to maintain a shared library alive for a very long time. It is going to resist lazy and conservative new builders and in addition fully-energized, radical and extremely opinionated ones. Modifications, enhancements or revolutionary refactors will likely be clean.
From a code perspective, ETLs ought to have 3 clearly differentiated high-level capabilities. Every one associated to one of many following steps: Extract, Remodel, Load. This is among the easiest necessities for ETL code.
def extract(supply: str) -> pd.DataFrame:
...
def rework(knowledge: pd.DataFrame) -> pd.DataFrame:
...
def load(transformed_data: pd.DataFrame):
...
Clearly, it’s not obligatory to call these capabilities like this, however it offers you a plus on readability as they’re broadly accepted phrases.
DRY (Don’t Repeat Your self)
This is among the nice design patterns which justifies a connectors library. You write it as soon as and reuse it throughout diferent steps or tasks.
Practical Programming
This can be a programming model that goals at making capabilities “pure” or with out side-effects. Inputs have to be immutable and outputs are all the time the identical given these inputs. These capabilities are simpler to check and debug in isolation. Subsequently, gives a greater diploma of reproducibility to knowledge pipelines.
With purposeful programming utilized to ETLs, we must always have the ability to present idempotency. Which means each time we run (or re-run) the pipeline, it ought to return the identical outputs. With this attribute, we’re capable of confidently function ETLs and make sure that double runs gained’t generate duplicate knowledge. What number of occasions you needed to create a bizarre SQL question to take away inserted rows from a incorrect ETL run? Making certain idempotency helps avoiding these conditions. Maxime Beauchemin, creator of Apache Airflow and Superset, is one recognized advocate for Practical Knowledge Engineering.
SOLID
We’ll use references to lessons definitions, however this part can be utilized to first-class capabilities. We will likely be utilizing heavy object-oriented programming to clarify these ideas, however it doesn’t imply that is the easiest way of growing an ETL. There’s not a particular consensus and every firm does it by itself method.
Relating to the Single Duty Precept, it’s essential to create entities which have just one cause to alter. For instance, segregating obligations amongst two objects resembling a SalesAggregator and a SalesDataCleaner class. The latter is prone to include particular enterprise guidelines to “clear” knowledge from gross sales, and the previous is targeted on extracting gross sales from disparate methods. Each lessons code can change due to totally different causes.
For the Open-Shut Precept, entities ought to be expandable so as to add new options however not opened to be modified. Think about that the SalesAggregator acquired as parts a StoresSalesCollector which is used to extract gross sales from bodily shops. If the corporate began promoting on-line and we wished to get that knowledge, we might state that SalesCollector is open for extension if it might probably obtain additionally one other OnlineSalesCollector with a suitable interface.
from abc import ABC, abstractmethod
class BaseCollector(ABC):
@abstractmethod
def extract_sales() -> Record[Sale]:
move
class SalesAggregator:
def __init__(self, collectors: Record[BaseCollector]):
self.collectors = collectors
def get_sales(self) -> Record[Sale]:
gross sales = []
for collector in self.collectors:
gross sales.prolong(collector.extract_sales())
return gross sales
class StoreSalesCollector:
def extract_sales() -> Record[Sale]:
# Extract gross sales knowledge from bodily shops
class OnlineSalesCollector:
def extract_sales() -> Record[Sale]:
# Extract on-line gross sales knowledge
if __name__ == "__main__":
sales_aggregator = SalesAggregator(
collectors = [
StoreSalesCollector(),
OnlineSalesCollector()
]
gross sales = sales_aggregator.get_sales()
The Liskov substitution precept, or behavioural subtyping isn’t so simple to use to ETL design, however it’s for the utilities library we talked about earlier than. This precept tries to set a rule for subtypes. In a given program that makes use of the supertype, one may potential substitute it with one subtype with out altering the behaviour of this system.
from abc import ABC, abstractmethod
class DatabaseConnector(ABC):
def __init__(self, connection_string: str):
self.connection_string = connection_string
@abstractmethod
def join():
move
@abstractmethod
def execute_(question: str) -> pd.DataFrame:
move
class RedshiftConnector(DatabaseConnector):
def join():
# Redshift Connection implementation
def execute(question: str) -> pd.DataFrame:
# Redshift Connection implementation
class BigQueryConnector(DatabaseConnector):
def join():
# BigQuery Connection implementation
def execute(question: str) -> pd.DataFrame:
# BigQuery Connection implementation
class ETLQueryManager:
def __init__(self, connector: DatabaseConnector, connection_string: str):
self.connector = connector(connection_string=connection_string).join()
def run(self, sql_queries: Record[str]):
for question in sql_queries:
self.connector.execute(question=question)
We see within the instance under that any of the DatabaseConnector subtypes conform to the Liskov substitution precept as any of its subtypes may very well be used inside the ETLManager class.
Now, let’s discuss in regards to the Interface Segregation Precept. It states that shoppers shouldn’t depend upon interfaces they don’t use. This one comes very helpful for the DatabaseConnector design. For those who’re implementing a DatabaseConnector, don’t overload the interface class with strategies that gained’t be used within the context of an ETL. For instance, you gained’t want strategies resembling grant_permissions, or check_log_errors. These are associated to an administrative utilization of the database, which isn’t the case.
The one however not least, the Dependency Inversion precept. This one says that high-level modules shouldn’t depend upon lower-level modules, however as a substitute on abstractions. This behaviour is clearly exemplified with the SalesAggregator above. Discover that its __init__ technique doesn’t depend upon concrete implementations of both StoreSalesCollector or OnlineSalesCollector. It mainly is dependent upon a BaseCollector interface.
We’ve closely depend on object-oriented lessons within the examples above to indicate methods wherein we are able to apply SOLID ideas to ETL jobs. However, there is no such thing as a common consensus of what’s the most effective code format and commonplace to comply with when constructing an ETL. It may take very totally different kinds and it tends to be extra an issue of getting an inside well-documented opinionated framework, as mentioned beforehand, relatively than making an attempt to give you a world commonplace throughout the {industry}.
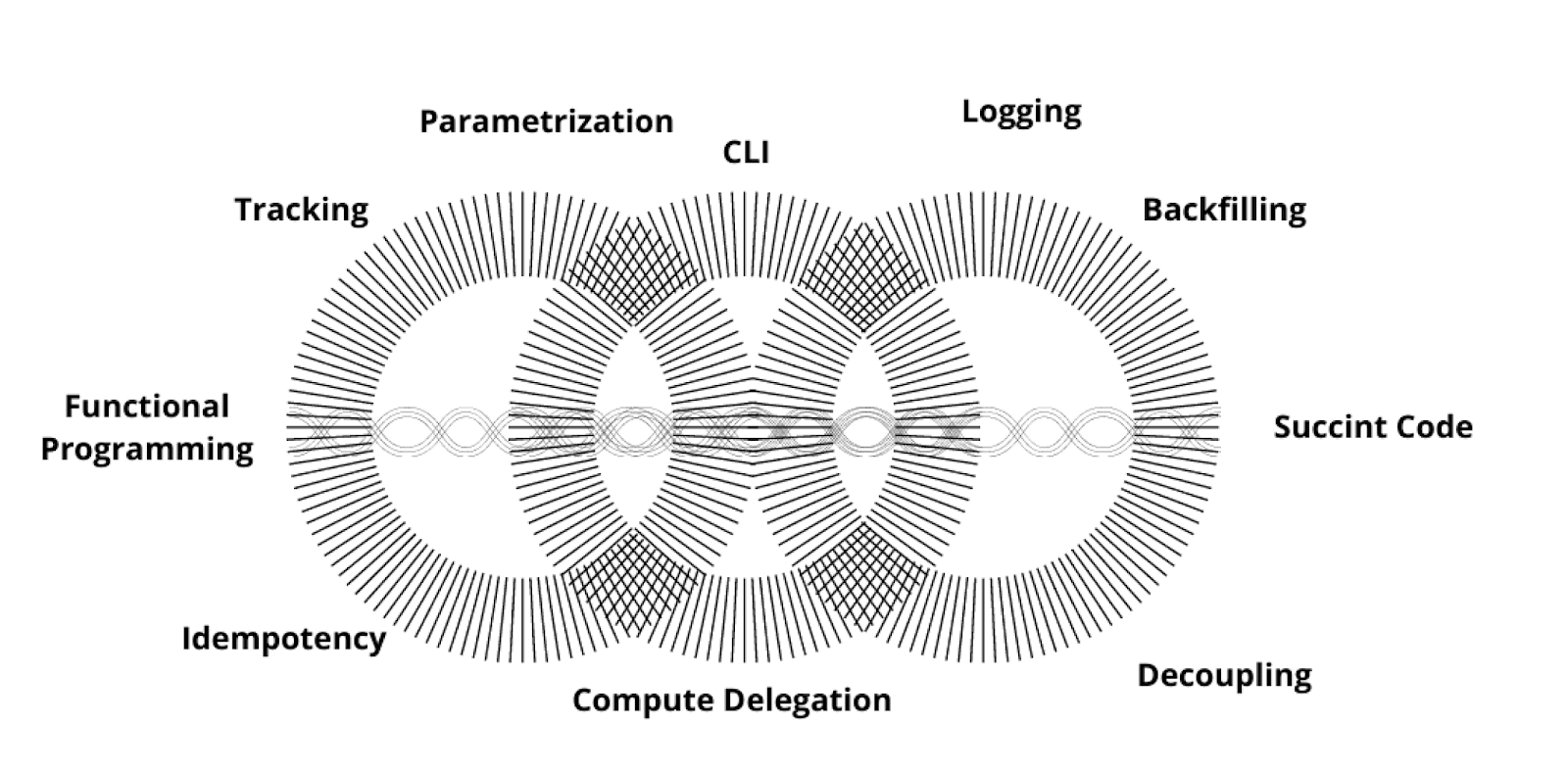
Therefore, on this part, I’ll attempt to concentrate on explaining some traits that make ETL code extra legible, safe and dependable.
Command Line Purposes
All Knowledge Processes that you can imagine are mainly command line purposes. When growing your ETL in Python, all the time present a parametrized CLI interface to be able to execute it from anywhere (E.g, a Docker container that may run underneath a Kubernetes cluster). There are a selection of instruments for constructing command-line arguments parsing resembling argparse, click on, typer, yaspin or docopt. Typer is presumably essentially the most versatile, simple to make use of an non-invasive to your present codebase. It was constructed by the creator of the well-known Python net companies library FastApi, and its Github begins continue to grow. The documentation is nice and is changing into increasingly more industry-standard.
from typer import Typer
app = Typer()
@app.command()
def run_etl(
atmosphere: str,
start_date: str,
end_date: str,
threshold: int
):
...
To run the above command, you’d solely must do:
python {file_name}.py run-etl --environment dev --start-date 2023/01/01 --end-date 2023/01/31 --threshold 10
Course of vs Database Engine Compute Commerce Off
The everyday suggestion when constructing ETLs on high of a Knowledge Warehouse is to push as a lot compute processing to the Knowledge Warehouse as potential. That’s all proper if in case you have a knowledge warehouse engine that autoscales based mostly on demand. However that’s not the case for each firm, state of affairs or crew. Some ML queries will be very lengthy and overload the cluster simply. It’s typical to mixture knowledge from very disparate tables, lookback for years of information, carry out point-in-time clauses, and so on. Therefore, pushing the whole lot to the cluster isn’t all the time the most suitable choice. Isolating the compute into the reminiscence of the method occasion will be safer in some instances. It’s risk-free as you gained’t hit the cluster and probably break or delay business-critical queries. That is an apparent state of affairs for Spark customers, as all of the compute & knowledge will get distributed throughout the executors due to the huge scale they want. However for those who’re working over Redshift or BigQuery clusters all the time hold a watch into how a lot compute you’ll be able to delegate to them.
Observe Outputs
ML ETLs generate several types of output artifacts. Some are Parquet information in HDFS, CSV information in S3, tables within the knowledge warehouse, mapping information, studies, and so on. These information can later be used to coach fashions, enrich knowledge in manufacturing, fetch options on-line and plenty of extra choices.
That is fairly useful as you’ll be able to hyperlink dataset constructing jobs with coaching jobs utilizing the identifier of the artifacts. For instance, when utilizing Neptune track_files() technique, you’ll be able to observe these form of information. There’s a really clear instance right here that you should use.
Implement Automated Backfilling
Think about you have got a day by day ETL that will get final day’s knowledge to compute a characteristic used to coach a mannequin If for any cause your ETL fails to run for a day, the following day it runs you’ll have misplaced the day past knowledge computed.
To resolve this, it’s a superb apply to have a look at what’s the final registered timestamp within the vacation spot desk or file. Then, the ETL will be executed for these lagging two days.
Develop Loosely Coupled Parts
Code could be very prone to alter, and processes that depend upon knowledge much more. Occasions that construct up tables can evolve, columns can change, sizes can improve, and so on. When you have got ETLs that depend upon totally different sources of knowledge is all the time good to isolate them within the code. It’s because if at any time it’s a must to separate each parts as two totally different duties (E.g: One wants a much bigger occasion sort to run the processing as a result of the information has grown), it’s a lot simpler to do if the code isn’t spaghetti!
Make Your ETLs Idempotent
It’s typical to run the identical course of greater than as soon as in case there was a problem on the supply tables or inside the course of itself. To keep away from producing duplicate knowledge outputs or half-filled tables, ETLs ought to be idempotent. That’s, for those who by accident run the identical ETL twice with the identical situations that the primary time, the output or side-effects from the primary run shouldn’t be affected (ref). You possibly can guarantee that is imposed in your ETL by making use of the delete-write sample, the pipeline will first delete the present knowledge earlier than writing new knowledge.
Hold Your ETLs Code Succinct
I all the time wish to have a transparent separation between the precise implementation code from the enterprise/logical layer. After I’m constructing an ETL, the primary layer ought to be learn as a sequence of steps (capabilities or strategies) that clearly state what is going on to the information. Having a number of layers of abstraction isn’t dangerous. It’s very useful if in case you have have to keep up the ETL for years.
All the time isolate high-level and low-level capabilities from one another. It is extremely bizarre to seek out one thing like:
from config import CONVERSION_FACTORS
def transform_data(knowledge: pd.DataFrame) -> pd.DataFrame:
knowledge = remove_duplicates(knowledge=knowledge)
knowledge = encode_categorical_columns(knowledge=knowledge)
knowledge["price_dollars"] = knowledge["price_euros"] * CONVERSION_FACTORS["dollar-euro"]
knowledge["price_pounds"] = knowledge["price_euros"] * CONVERSION_FACTORS["pound-euro"]
return knowledge
Within the instance above we’re utilizing high-level capabilities such because the “remove_duplicates” and “encode_categorical_columns” however on the identical time we’re explicitly exhibiting an implementation operation to transform the value with a conversion issue. Wouldn’t it’s nicer to take away these 2 strains of code and substitute them with a “convert_prices” perform?
from config import CONVERSION_FACTOR
def transform_data(knowledge: pd.DataFrame) -> pd.DataFrame:
knowledge = remove_duplicates(knowledge=knowledge)
knowledge = encode_categorical_columns(knowledge=knowledge)
knowledge = convert_prices(knowledge=knowledge)
return knowledge
On this instance, readability wasn’t an issue, however think about that as a substitute, you embed a 5 strains lengthy groupby operation within the “transform_data” together with the “remove_duplicates” and “encode_categorical_columns”. In each instances, you’re mixing high-level and low-level capabilities. It’s extremely really useful to maintain a cohesive layered code. Generally is inevitable and over-engineered to maintain a perform or module 100% cohesively layered, however it’s a really useful purpose to pursue.
Use Pure Features
Don’t let side-effects or international states complicate your ETLs. Pure capabilities return the identical outcomes if the identical arguments are handed.
❌The perform under isn’t pure. You’re passing a dataframe that’s joined with one other capabilities that’s learn from an out of doors supply. Which means the desk can change, therefore, returning a unique dataframe, probably, every time the perform is known as with the identical arguments.
def transform_data(knowledge: pd.DataFrame) -> pd.DataFrame:
reference_data = read_reference_data(desk="public.references")
knowledge = knowledge.be a part of(reference_data, on="ref_id")
return knowledge
To make this perform pure, you would need to do the next:
def transform_data(knowledge: pd.DataFrame, reference_data: pd.DataFrame) -> pd.DataFrame:
knowledge = knowledge.be a part of(reference_data, on="ref_id")
return knowledge
Now, when passing the identical “knowledge” and “reference_data” arguments, the perform will yield the identical outcomes.
This can be a easy instance, however all of us have witnessed worse conditions. Features that depend on international state variables, strategies that change the state of sophistication attributes based mostly on sure situations, probably altering the behaviour of different upcoming strategies within the ETL, and so on.
Maximising the usage of pure capabilities results in extra purposeful ETLs. As we’ve already mentioned in factors above, it comes with nice advantages.
Paremetrize As A lot As You Can
ETLs change. That’s one thing that we’ve to imagine. Supply desk definitions change, enterprise guidelines change, desired outcomes evolve, experiments are refined, ML fashions require extra subtle options, and so on.
With a view to have some extent of flexibility in our ETLs, we have to totally assess the place to place a lot of the effort to supply parametrised executions of the ETLs. Parametrisation is a attribute wherein, simply by altering parameters by means of a easy interface, we are able to alter the behaviour of the method. The interface is usually a YAML file, a category initialisation technique, perform arguments and even CLI arguments.
A easy simple parametrisation is to outline the “atmosphere”, or “stage” of the ETL. Earlier than working the ETL into manufacturing, the place it might probably have an effect on downstream processes and methods, it’s good to have a “check”, “integration” or “dev” remoted environments in order that we are able to check our ETLs. That atmosphere may contain totally different ranges of isolation. It may go from the execution infrastructure (dev cases remoted from manufacturing cases), object storage, knowledge warehouse, knowledge sources, and so on.
That’s an apparent parameter and doubtless crucial. However we are able to broaden the parametrisation additionally to business-related arguments. We will parametrise window dates to run the ETL, columns names that may change or be refined, knowledge sorts, filtering values, and so on.
Simply The Proper Quantity Of Logging
This is among the most underestimated properties of an ETL. Logs are helpful to detect manufacturing executions anomalies or implicit bugs or clarify knowledge units. It’s all the time helpful to log properties about extracted knowledge. Other than in-code validations to make sure the totally different ETL steps run efficiently, we are able to additionally log:
- References to supply tables, APIs or vacation spot paths (E.g: “Getting knowledge from `item_clicks` desk”)
- Modifications in anticipated schemas (E.g: “There’s a new column in `promotion` desk”)
- The variety of rows fetched (E.g: “Fetched 145234093 rows from `item_clicks` desk”)
- The variety of null values in crucial columns (E.g: “Discovered 125 null values in Supply column”)
- Easy statistics of information (e.g: imply, commonplace deviation, and so on). (E.g: “CTR imply: 0.13, CTR std: 0.40)
- Distinctive values for categorical columns (E.g: “Nation column contains: ‘Spain’, ‘France’ and ‘Italy’”)
- Variety of rows deduplicated (E.g: “Eliminated 1400 duplicated rows”)
- Execution occasions for compute-intensive operations (E.g: “Aggregation took 560s”)
- Completion checkpoints for various phases of the ETL (e.g: “Enrichment course of completed efficiently”)
Manuel Martín is an Engineering Supervisor with greater than 6 years of experience in knowledge science. He have beforehand labored as a knowledge scientist and a machine studying engineer and now I lead the ML/AI apply at Busuu.