
Picture by Creator
Newbie programmers take pleasure in coding in Python due to its simplicity and easy-to-read syntax. Writing environment friendly Python code, nonetheless, is extra concerned than you assume. It requires understanding of a few of the options of the language (they’re simply as easy to choose up, although).
For those who’re coming from one other programming language similar to C++ or JavaScript, this tutorial is so that you can be taught some tricks to write environment friendly code in Python. However in case you are a newbie—studying Python as your first (programming) language—then this tutorial will assist you to write Pythonic code from the get go.
We’ll deal with the next:
- Pythonic loops
- Listing and dictionary comprehension
- Context managers
- Turbines
- Assortment lessons
So let’s dive in!
Understanding loop constructs is essential whatever the language you’re programming in. For those who’re coming from languages similar to C++ or JavaScript, it is useful to discover ways to write Pythonic loops.
Generate a Sequence of Numbers with vary
The vary()
operate generates a sequence of numbers, typically used as an iterator in loops.
The vary()
operate returns a spread object that begins from 0 by default and goes as much as (however would not embody) the required quantity.
Right here’s an instance:
for i in vary(5):
print(i)
When utilizing the vary()
operate, you possibly can customise the place to begin, ending level, and step dimension as wanted.
Entry Each Index and Merchandise with enumerate
The enumerate()
operate is beneficial once you need each the index and the worth of every aspect in an iterable.
On this instance, we use the index to faucet into the fruits
listing:
fruits = ["apple", "banana", "cherry"]
for i in vary(len(fruits)):
print(f"Index {i}: {fruits[i]}")
Output >>>
Index 0: apple
Index 1: banana
Index 2: cherry
However with the enumerate()
operate, you possibly can entry each the index and the aspect like so:
fruits = ["apple", "banana", "cherry"]
for i, fruit in enumerate(fruits):
print(f"Index {i}: {fruit}")
Output >>>
Index 0: apple
Index 1: banana
Index 2: cherry
Iterate in Parallel Over A number of Iterables with zip
The zip()
operate is used to iterate over a number of iterables in parallel. It pairs corresponding parts from completely different iterables collectively.
Take into account the next instance the place it is advisable to loop by way of each names
and scores
listing:
names = ["Alice", "Bob", "Charlie"]
scores = [95, 89, 78]
for i in vary(len(names)):
print(f"{names[i]} scored {scores[i]} factors.")
This outputs:
Output >>>
Alice scored 95 factors.
Bob scored 89 factors.
Charlie scored 78 factors.
This is a way more readable loop with the zip()
operate:
names = ["Alice", "Bob", "Charlie"]
scores = [95, 89, 78]
for title, rating in zip(names, scores):
print(f"{title} scored {rating} factors.")
Output >>>
Alice scored 95 factors.
Bob scored 89 factors.
Charlie scored 78 factors.
The Pythonic model utilizing zip()
is extra elegant and avoids the necessity for guide indexing—making the code cleaner and extra readable.
In Python, listing comprehensions and dictionary comprehensions are concise one-liners to create lists and dictionaries, respectively. They’ll additionally embody conditional statements to filter gadgets primarily based on sure circumstances.
Let’s begin with the loop model after which transfer on to comprehension expressions for each lists and dictionaries.
Listing Comprehension in Python
Say you may have a numbers
listing. And also you’d prefer to create a squared_numbers
listing. You should utilize a for loop like so:
numbers = [1, 2, 3, 4, 5]
squared_numbers = []
for num in numbers:
squared_numbers.append(num ** 2)
print(squared_numbers)
Output >>> [1, 4, 9, 16, 25]
However listing comprehensions present a cleaner and less complicated syntax to do that. They assist you to create a brand new listing by making use of an expression to every merchandise in an iterable.
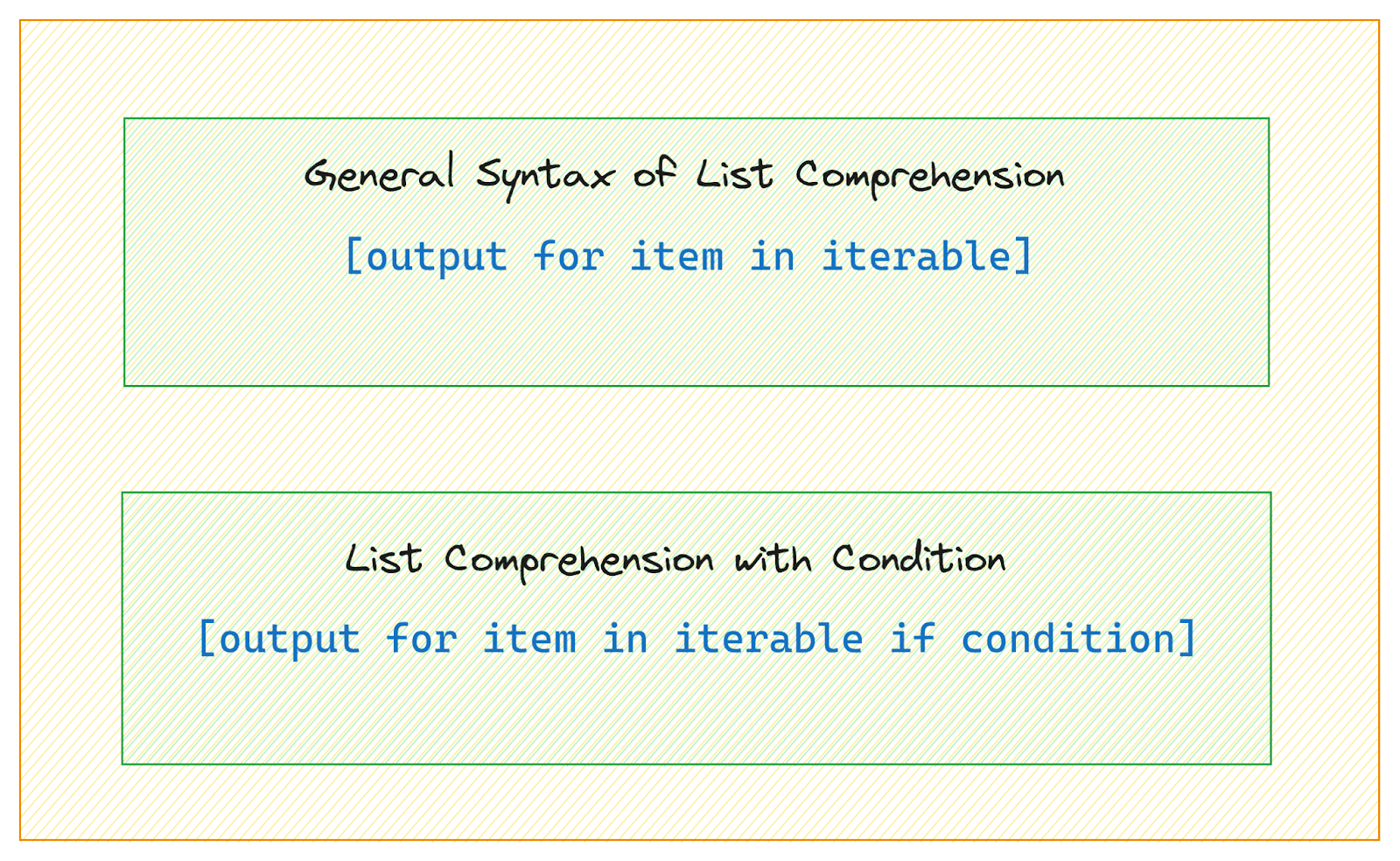
Listing Comprehension Syntax | Picture by Creator
Right here’s a concise different utilizing a listing comprehension expression:
numbers = [1, 2, 3, 4, 5]
squared_numbers = [num ** 2 for num in numbers]
print(squared_numbers)
Output >>> [1, 4, 9, 16, 25]
Right here, the listing comprehension creates a brand new listing containing the squares of every quantity within the numbers
listing.
Listing Comprehension with Conditional Filtering
You can too add filtering circumstances inside the listing comprehension expression. Take into account this instance:
numbers = [1, 2, 3, 4, 5]
odd_numbers = [num for num in numbers if num % 2 != 0]
print(odd_numbers)
On this instance, the listing comprehension creates a brand new listing containing solely the odd numbers from the numbers
listing.
Dictionary Comprehension in Python
With a syntax just like listing comprehension, dictionary comprehension means that you can create dictionaries from current iterables.
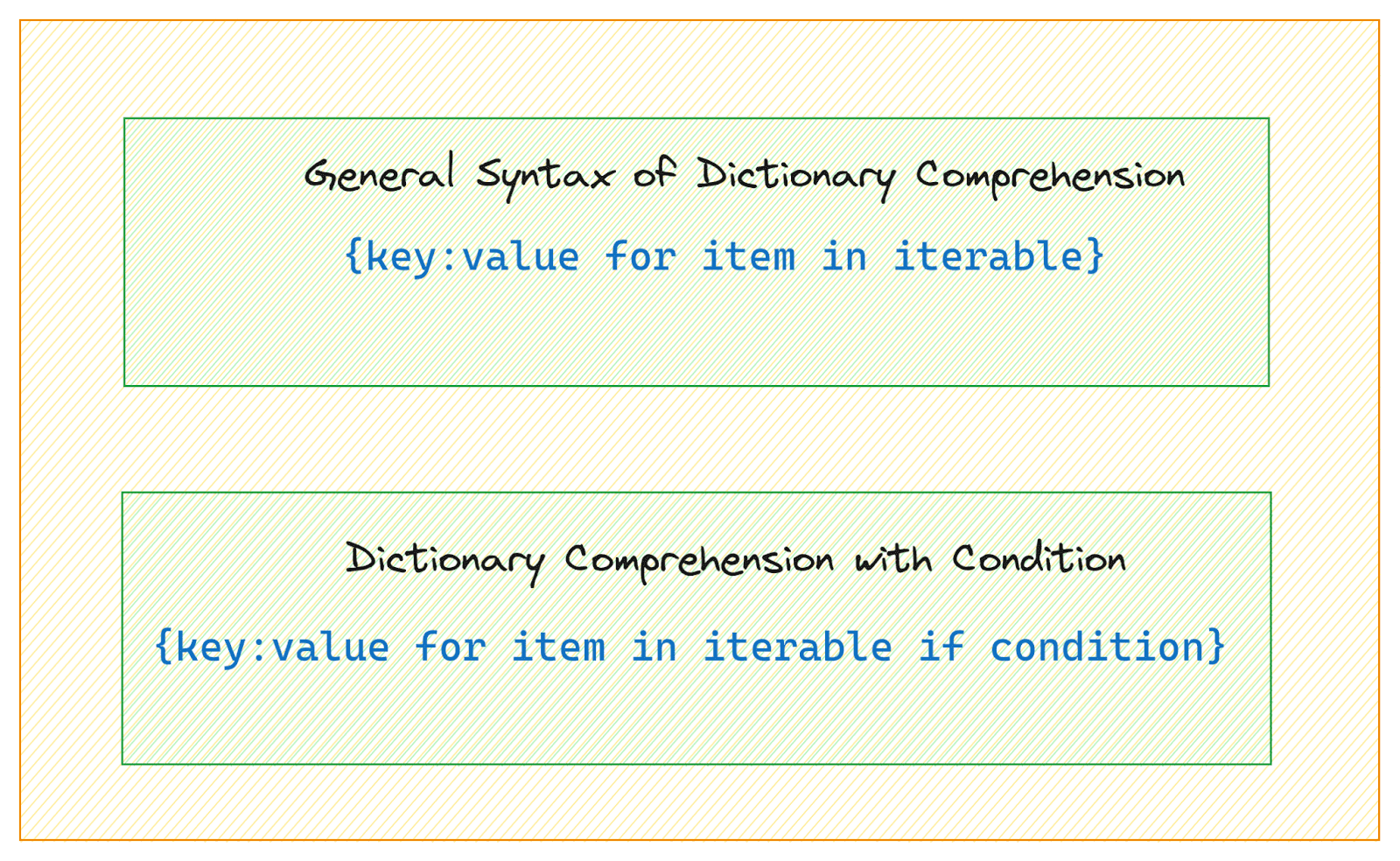
Dictionary Comprehension Syntax | Picture by Creator
Say you may have a fruits
listing. You’d prefer to create a dictionary with fruit:len(fruit)
key-value pairs.
Right here’s how you are able to do this with a for loop:
fruits = ["apple", "banana", "cherry", "date"]
fruit_lengths = {}
for fruit in fruits:
fruit_lengths[fruit] = len(fruit)
print(fruit_lengths)
Output >>> {'apple': 5, 'banana': 6, 'cherry': 6, 'date': 4}
Let’s now write the dictionary comprehension equal:
fruits = ["apple", "banana", "cherry", "date"]
fruit_lengths = {fruit: len(fruit) for fruit in fruits}
print(fruit_lengths)
Output >>> {'apple': 5, 'banana': 6, 'cherry': 6, 'date': 4}
This dictionary comprehension creates a dictionary the place keys are the fruits and values are the lengths of the fruit names.
Dictionary Comprehension with Conditional Filtering
Let’s modify our dictionary comprehension expression to incorporate a situation:
fruits = ["apple", "banana", "cherry", "date"]
long_fruit_names = {fruit: len(fruit) for fruit in fruits if len(fruit) > 5}
print(long_fruit_names)
Output >>> {'banana': 6, 'cherry': 6}
Right here, the dictionary comprehension creates a dictionary with fruit names as keys and their lengths as values, however just for fruits with names longer than 5 characters.
Context managers in Python assist you to handle sources effectively. With context managers, you possibly can arrange and tear down (clear up) sources simply. The best and the commonest instance of context managers is in file dealing with.
Have a look at the code snippet under:
filename="somefile.txt"
file = open(filename,'w')
file.write('One thing')
It would not shut the file descriptor leading to useful resource leakage.
print(file.closed)
Output >>> False
You’ll most likely provide you with the next:
filename="somefile.txt"
file = open(filename,'w')
file.write('One thing')
file.shut()
Whereas this makes an attempt to shut the descriptor, it doesn’t account for the errors that will come up through the write operation.
Properly, it’s possible you’ll now implement exception dealing with to attempt to open a file and write one thing within the absence of any errors:
filename="somefile.txt"
file = open(filename,'w')
attempt:
file.write('One thing')
lastly:
file.shut()
However that is verbose. Now have a look at the next model utilizing the with
assertion that helps open()
operate which is a context supervisor:
filename="somefile.txt"
with open(filename, 'w') as file:
file.write('One thing')
print(file.closed)
We use the with
assertion to create a context by which the file is opened. This ensures that the file is correctly closed when the execution exits the with
block—even when an exception is raised through the operation.
Turbines present a sublime approach to work with giant datasets or infinite sequences—bettering code effectivity and decreasing reminiscence consumption.
What Are Turbines?
Turbines are capabilities that use the yield
key phrase to return values separately, preserving their inside state between invocations. Not like common capabilities that compute all values directly and return a whole listing, mills compute and yield values on-the-fly as they’re requested, making them appropriate for processing giant sequences.
How Do Turbines Work?
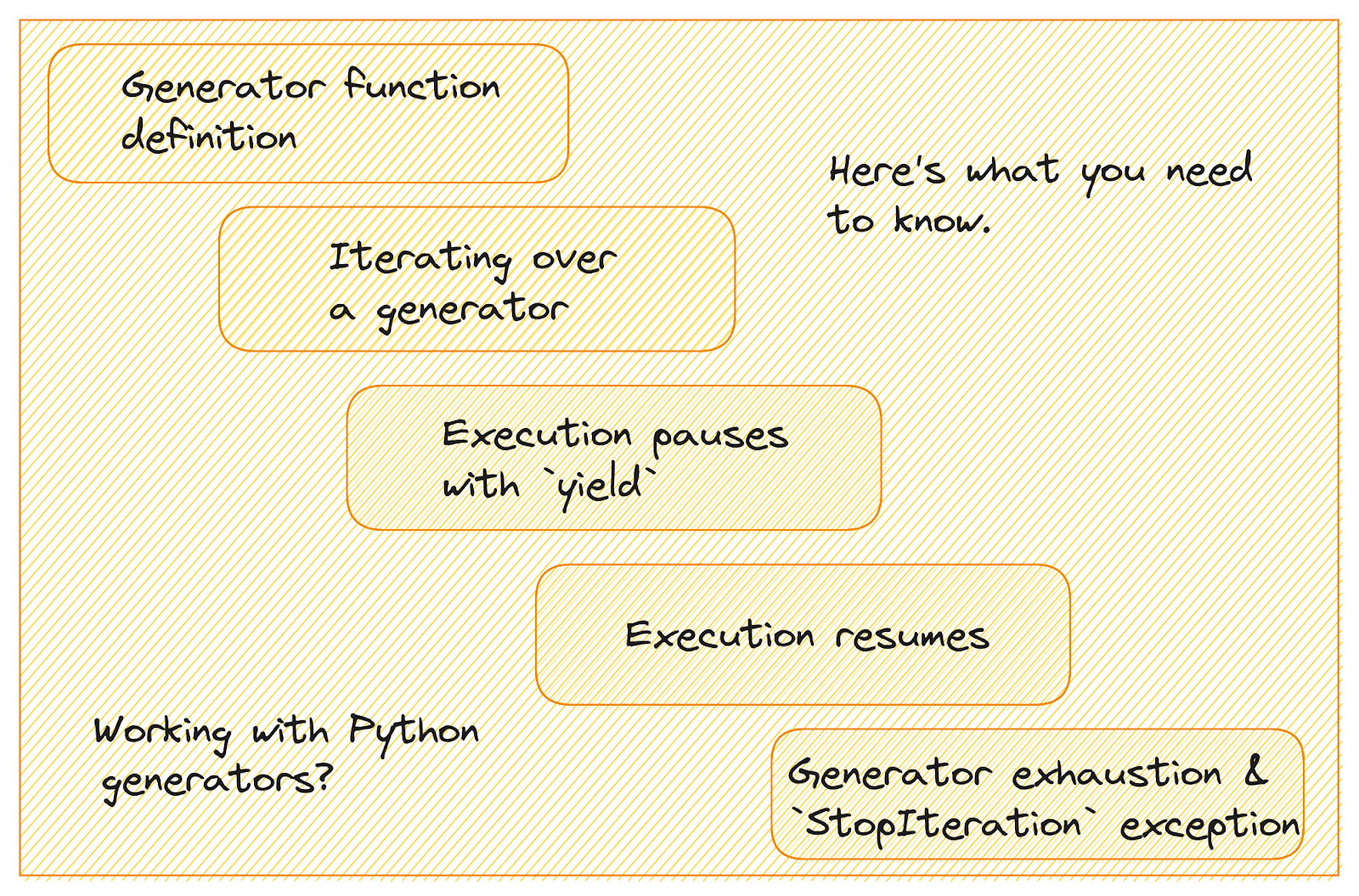
Picture by Creator
Let’s find out how mills work:
- A generator operate is outlined like a daily operate, however as a substitute of utilizing the
return
key phrase, you’ll useyield
to yield a worth. - Once you name a generator operate, it returns a generator object. Which you’ll be able to iterate over utilizing a loop or by calling
subsequent()
. - When the
yield
assertion is encountered, the operate’s state is saved, and the yielded worth is returned to the caller. The operate’s execution pauses, however its native variables and state are retained. - When the generator’s
subsequent()
methodology known as once more, execution resumes from the place it was paused, and the operate continues till the subsequentyield
assertion. - When the operate exits or raises a
StopIteration
exception, the generator is taken into account exhausted, and additional calls tosubsequent()
will increaseStopIteration
.
Creating Turbines
You’ll be able to create mills utilizing both generator capabilities or generator expressions.
Right here’s an instance generator operate:
def countdown(n):
whereas n > 0:
yield n
n -= 1
# Utilizing the generator operate
for num in countdown(5):
print(num)
Generator expressions are just like listing comprehension however they create mills as a substitute of lists.
# Generator expression to create a sequence of squares
squares = (x ** 2 for x in vary(1, 6))
# Utilizing the generator expression
for sq. in squares:
print(sq.)
We’ll wrap up the tutorial by studying about two helpful assortment lessons:
Extra Readable Tuples with NamedTuple
In Python, a namedtuple within the collections module is a subclass of the built-in tuple class. However it offers named fields. Which makes it extra readable and self-documenting than common tuples.
Right here’s an instance of making a easy tuple for a degree in 3D area and accessing the person parts:
# 3D level tuple
coordinate = (1, 2, 3)
# Accessing knowledge utilizing tuple unpacking
x, y, z = coordinate
print(f"X-coordinate: {x}, Y-coordinate: {y}, Z-coordinate: {z}")
Output >>> X-coordinate: 1, Y-coordinate: 2, Z-coordinate: 3
And right here’s the namedtuple model:
from collections import namedtuple
# Outline a Coordinate3D namedtuple
Coordinate3D = namedtuple("Coordinate3D", ["x", "y", "z"])
# Making a Coordinate3D object
coordinate = Coordinate3D(1, 2, 3)
print(coordinate)
# Accessing knowledge utilizing named fields
print(f"X-coordinate: {coordinate.x}, Y-coordinate: {coordinate.y}, Z-coordinate: {coordinate.z}")
Output >>>
Coordinate3D(x=1, y=2, z=3)
X-coordinate: 1, Y-coordinate: 2, Z-coordinate: 3
NamedTuples, subsequently, allow you to write cleaner and extra maintainable code than common tuples.
Use Counter to Simplify Counting
Counter is a category within the collections module that’s designed for counting the frequency of parts in an iterable similar to a listing or a string). It returns a Counter object with {aspect:rely}
key-value pairs.
Let’s take the instance of counting character frequencies in an extended string.
Right here’s the standard strategy to counting character frequencies utilizing loops:
phrase = "incomprehensibilities"
# initialize an empty dictionary to rely characters
char_counts = {}
# Rely character frequencies
for char in phrase:
if char in char_counts:
char_counts[char] += 1
else:
char_counts[char] = 1
# print out the char_counts dictionary
print(char_counts)
# discover the commonest character
most_common = max(char_counts, key=char_counts.get)
print(f"Most Widespread Character: '{most_common}' (seems {char_counts[most_common]} occasions)")
We manually iterate by way of the string, replace a dictionary to rely character frequencies, and discover the commonest character.
Output >>>
{'i': 5, 'n': 2, 'c': 1, 'o': 1, 'm': 1, 'p': 1, 'r': 1, 'e': 3, 'h': 1, 's': 2, 'b': 1, 'l': 1, 't': 1}
Most Widespread Character: 'i' (seems 5 occasions)
Now, let’s obtain the identical process utilizing the Counter class utilizing the syntax Counter(iterable)
:
from collections import Counter
phrase = "incomprehensibilities"
# Rely character frequencies utilizing Counter
char_counts = Counter(phrase)
print(char_counts)
# Discover the commonest character
most_common = char_counts.most_common(1)
print(f"Most Widespread Character: '{most_common[0][0]}' (seems {most_common[0][1]} occasions)")
Output >>>
Counter({'i': 5, 'e': 3, 'n': 2, 's': 2, 'c': 1, 'o': 1, 'm': 1, 'p': 1, 'r': 1, 'h': 1, 'b': 1, 'l': 1, 't': 1})
Most Widespread Character: 'i' (seems 5 occasions)
So Counter
offers a a lot less complicated approach to rely character frequencies with out the necessity for guide iteration and dictionary administration.
I hope you discovered just a few helpful ideas so as to add to your Python toolbox. In case you are seeking to be taught Python or are making ready for coding interviews, listed here are a few sources that will help you in your journey:
Completely happy studying!
Bala Priya C is a developer and technical author from India. She likes working on the intersection of math, programming, knowledge science, and content material creation. Her areas of curiosity and experience embody DevOps, knowledge science, and pure language processing. She enjoys studying, writing, coding, and occasional! Presently, she’s engaged on studying and sharing her data with the developer group by authoring tutorials, how-to guides, opinion items, and extra.