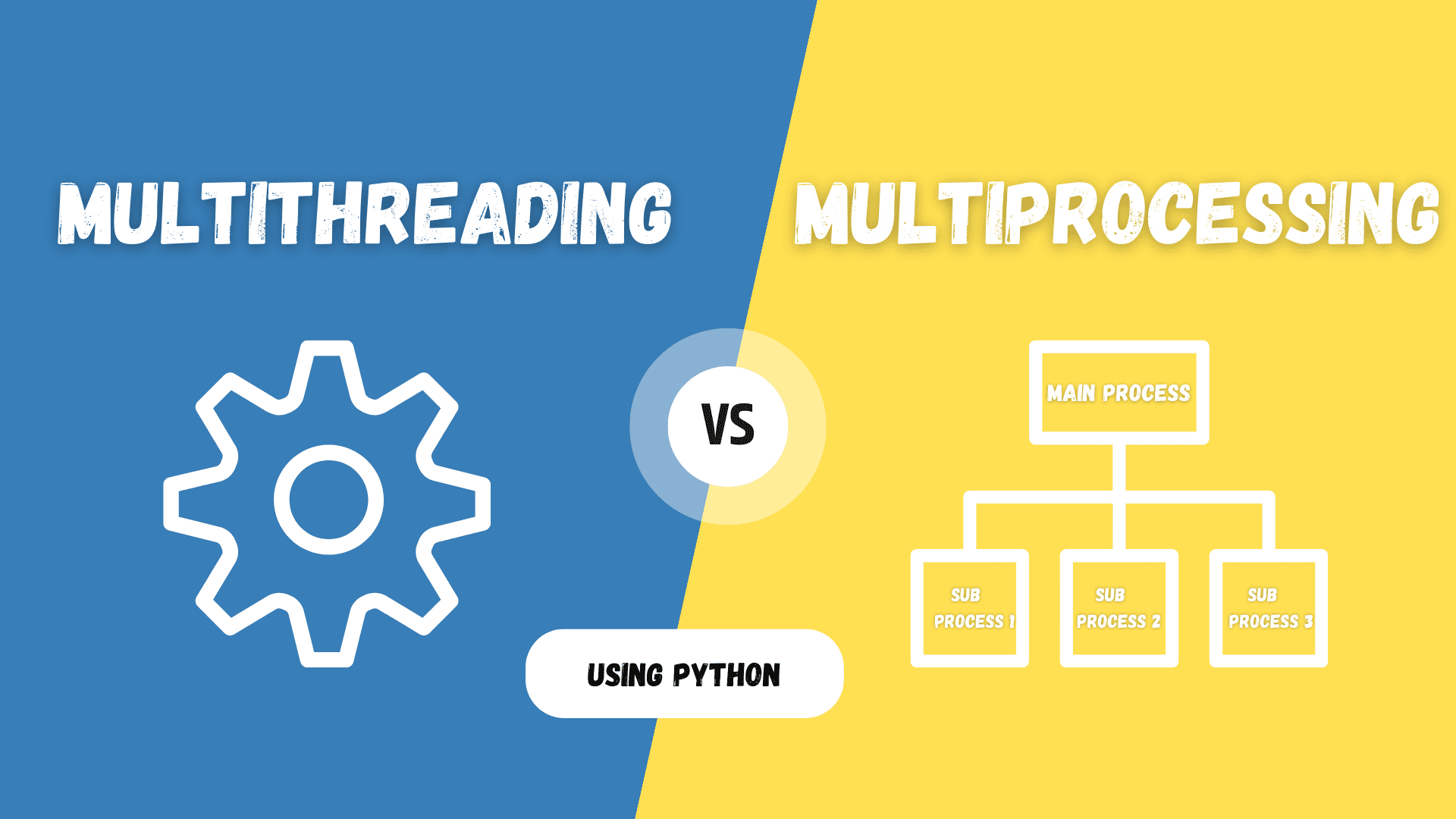
Picture by Creator
This tutorial will focus on leveraging Python’s functionality to execute multithreading and multiprogramming duties. They provide a gateway to carry out concurrent operations inside a single course of or throughout a number of processes. Parallel and concurrent execution will increase the velocity and effectivity of the techniques. After discussing the fundamentals of multithreading and multiprogramming, we may also focus on their sensible implementation utilizing Python libraries. Let’s first briefly focus on the advantages of parallel techniques.
- Improved Efficiency: With the potential to carry out duties concurrently, we are able to cut back the execution time and enhance the system’s total efficiency.
- Scalability: We will divide a big job into varied smaller sub-tasks and assign a separate core or thread to them for his or her impartial execution. It may be useful in large-scale techniques.
- Environment friendly I/O Operations: With the assistance of concurrency, the CPU doesn’t have to attend for a course of to finish its I/O operations. The CPU can instantly begin executing the next course of till the earlier course of is busy with its I/O.
- Useful resource Optimization: By dividing the sources, we are able to forestall a single course of from taking over all of the sources. This could keep away from the issue of Hunger for smaller processes.
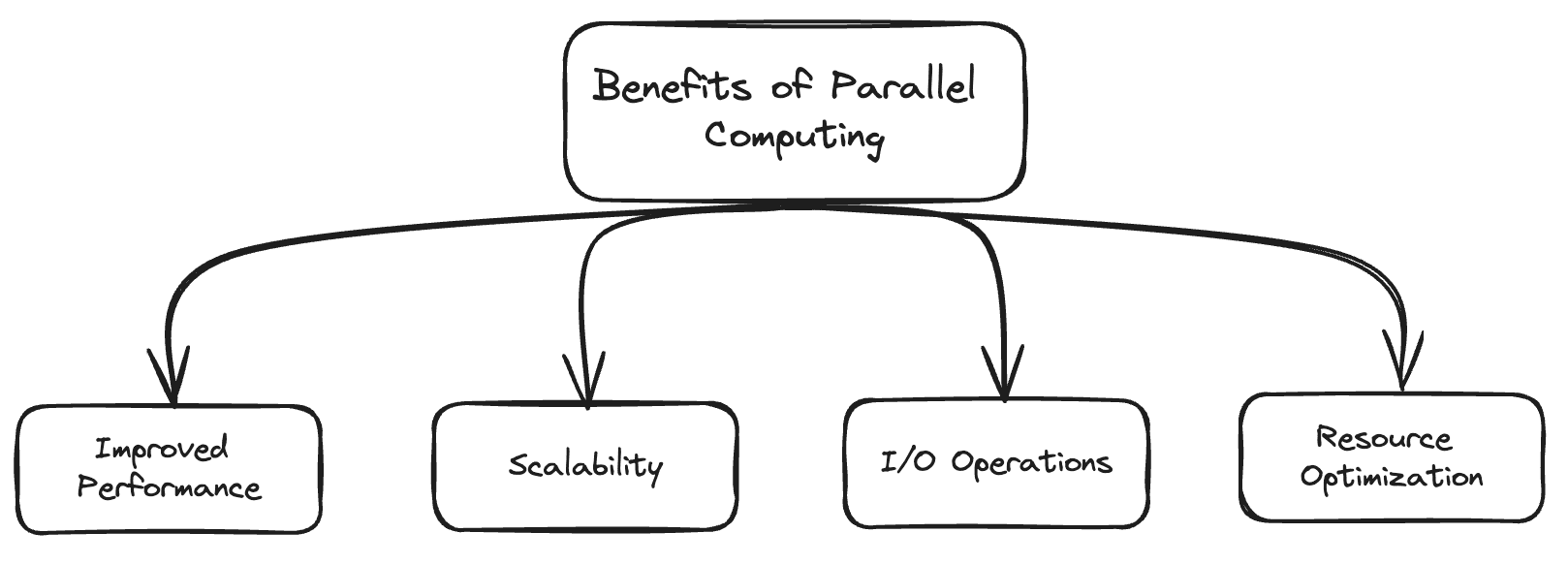
Advantages of Parallel Computing | Picture by Creator
These are some widespread causes for which you require concurrent or parallel executions. Now, transfer again to the principle matters, i.e., Multithreading and Multiprogramming, and focus on their major variations.
Multithreading is among the methods to realize parallelism in a single course of and capable of execute simultaneous duties. A number of threads might be created inside a single course of and carry out smaller duties parallel inside that course of.
The threads current inside a single course of share a standard reminiscence house, however their stack traces and registers are separate. They’re much less computationally costly attributable to this shared reminiscence.
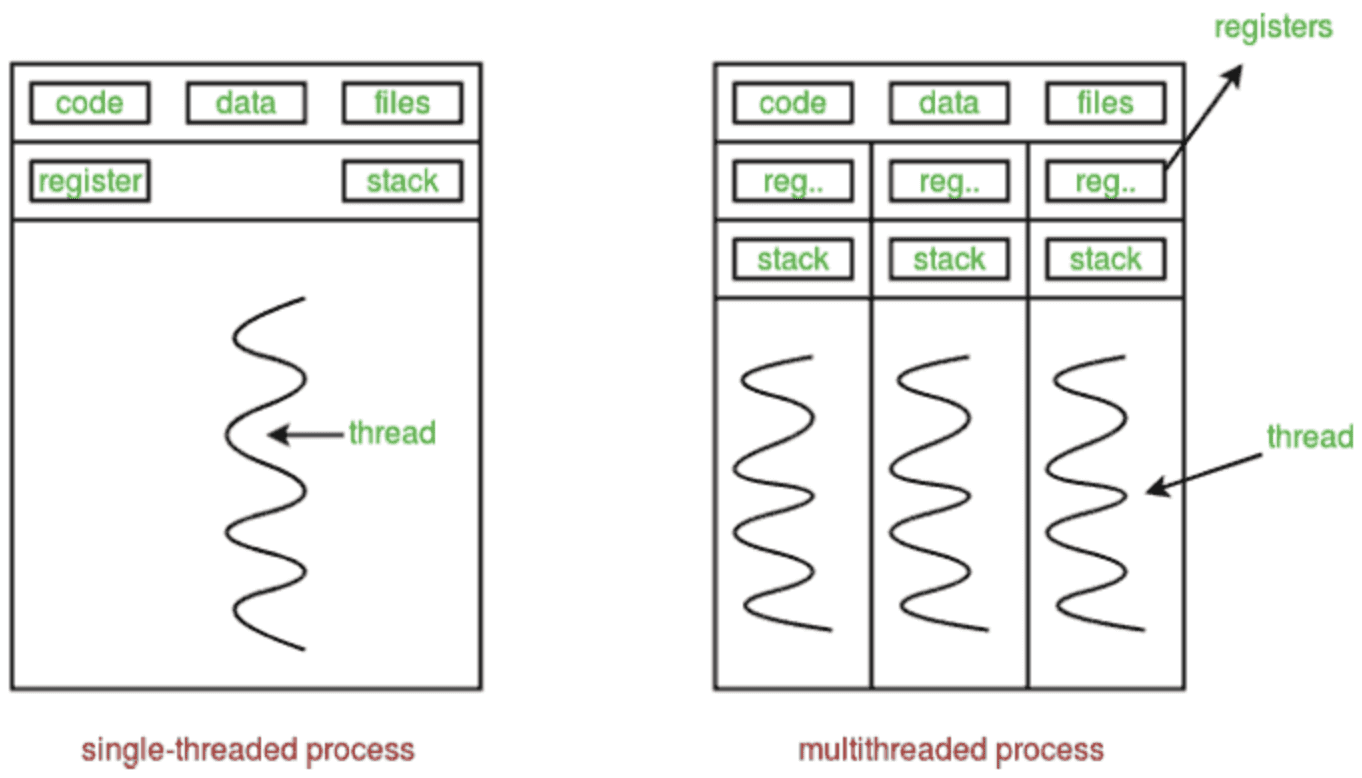
Single Threaded & Multi Threaded Env. | Picture by GeeksForGeeks
Multithreading is primarily utilized in performing I/O operations, i.e., if some a part of this system is busy in I/O operations, then the remaining program might be responsive. Nonetheless, in Python’s implementation, multithreading can’t obtain true parallelism attributable to World Interpreter Lock (GIL).
Briefly, GIL is a mutex lock that enables just one thread at a time to work together with the Python bytecode, i.e., even within the multithreaded mode, just one thread can execute the bytecode at a time.
It’s executed to take care of thread security in CPython, however this limits the efficiency advantages of multithreading. To handle this problem, python has a separate multiprocessing library, which we’ll focus on afterward.
What are Daemon Threads?
The threads which continuously run within the background are referred to as the demon threads. Their important job is to assist the principle thread or the non-daemon threads. The daemon thread doesn’t block the principle thread from execution and even retains operating if it has accomplished its execution.
In Python, the daemon threads are primarily used as a rubbish collector. It’s going to destroy all of the ineffective objects and free the reminiscence by default in order that the principle thread can be utilized and executed correctly.
Multiprocessing is used to carry out the parallel execution of a number of processes. It helps us obtain true parallelism, as we execute separate processes concurrently, having their very own reminiscence house. It makes use of separate cores of the CPU and can also be useful in performing inter-process communication to alternate information between a number of processes.
Multiprocessing is extra computationally costly as in comparison with multithreading, as we’re not utilizing a shared reminiscence house. Nonetheless, it permits us for impartial execution and overcomes World Interpreter Lock’s limitations.
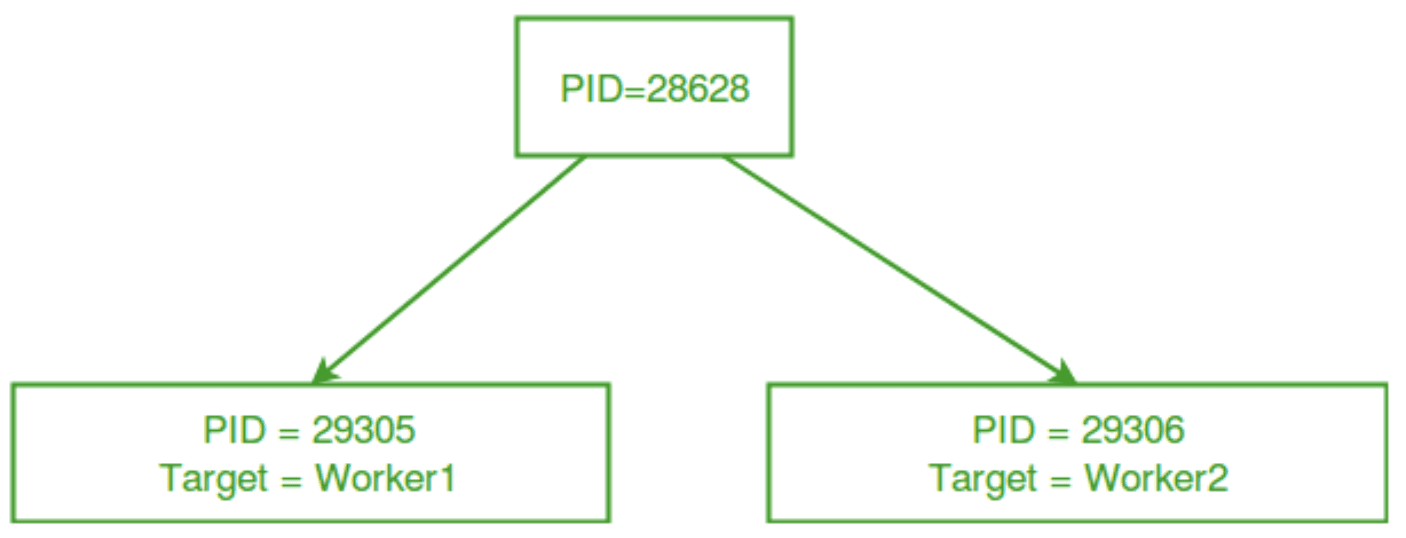
Multiprocessing Atmosphere | Picture by GeeksForGeeks
The above determine demonstrates a multi-processing atmosphere during which a important course of creates two separate processes and assigns separate work to them.
It’s time to implement a fundamental instance of multithreading utilizing Python. Python has an inbuilt module threading
used for the multithreading implementation.
- Importing Libraries:
import threading
import os
- Operate to Calculate the Squares:
It is a easy perform used to seek out the sq. of numbers. A listing of numbers is given as enter, and it outputs the sq. of every variety of the record together with the title of the thread used and the method ID related to that thread.
def calculate_squares(numbers):
for num in numbers:
sq. = num * num
print(
f"Sq. of the quantity {num} is {sq.} | Thread Title {threading.current_thread().title} | PID of the method {os.getpid()}"
)
- Foremost Operate:
We’ve a listing of numbers and we’ll divide that record equally and title them as fisrt_half and second_half
respectively. Now we’ll assign two separate threads t1
and t2
to those lists.
Thread
perform creates a brand new thread, which takes a perform with a listing of arguments to that perform. You too can assign a separate title to a thread.
.begin()
perform will begin executing these threads and .be a part of()
perform will block the execution of the principle thread till the given thread just isn’t executed utterly.
if __name__ == "__main__":
numbers = [1, 2, 3, 4, 5, 6, 7, 8]
half = len(numbers) // 2
first_half = numbers[:half]
second_half = numbers[half:]
t1 = threading.Thread(goal=calculate_squares, title="t1", args=(first_half,))
t2 = threading.Thread(goal=calculate_squares, title="t2", args=(second_half,))
t1.begin()
t2.begin()
t1.be a part of()
t2.be a part of()
Output:
Sq. of the number one is 1 | Thread Title t1 | PID of the method 345
Sq. of the quantity 2 is 4 | Thread Title t1 | PID of the method 345
Sq. of the quantity 5 is 25 | Thread Title t2 | PID of the method 345
Sq. of the quantity 3 is 9 | Thread Title t1 | PID of the method 345
Sq. of the quantity 6 is 36 | Thread Title t2 | PID of the method 345
Sq. of the quantity 4 is 16 | Thread Title t1 | PID of the method 345
Sq. of the quantity 7 is 49 | Thread Title t2 | PID of the method 345
Sq. of the quantity 8 is 64 | Thread Title t2 | PID of the method 345
Notice: All of the threads created above are non-daemon threads. To create a daemon thread, it is advisable to write
t1.setDaemon(True)
to make the threadt1
a daemon thread.
Now, we’ll perceive the output generated by the above code. We will observe that the method ID (i.e., PID) will stay the identical for each threads, which implies that these two threads are a part of the identical course of.
You too can observe that the output just isn’t generated sequentially. Within the first line, you will notice the output generated by thread1, then within the third line, the output generated by thread2, then once more by thread1 within the fourth line. This clearly signifies that these threads work collectively concurrently.
Concurrency doesn’t imply these two threads are executed parallelly, as just one thread is executed at a time. It doesn’t cut back the execution time. It takes the identical time as sequential execution. CPU begins executing a thread however leaves it halfway and strikes to a different thread, and after a while, comes again to the principle thread and begins its execution from the identical level it left final time.
I hope you will have a fundamental understanding of multithreading with its implementation and its limitations. Now, it’s time to study multiprocessing implementation and the way we are able to overcome these limitations.
We’ll comply with the identical instance, however as an alternative of making two separate threads, we’ll create two impartial processes and focus on the observations.
- Importing Libraries:
from multiprocessing import Course of
import os
We’ll use the multiprocessing
module to create impartial processes.
- Operate to Calculate the Squares:
That perform will stay the identical. We’ve simply eliminated the print assertion of threading info.
def calculate_squares(numbers):
for num in numbers:
sq. = num * num
print(
f"Sq. of the quantity {num} is {sq.} | PID of the method {os.getpid()}"
)
- Foremost Operate:
There are just a few modifications in the principle perform. We’ve simply created a separate course of as an alternative of a thread.
if __name__ == "__main__":
numbers = [1, 2, 3, 4, 5, 6, 7, 8]
half = len(numbers) // 2
first_half = numbers[:half]
second_half = numbers[half:]
p1 = Course of(goal=calculate_squares, args=(first_half,))
p2 = Course of(goal=calculate_squares, args=(second_half,))
p1.begin()
p2.begin()
p1.be a part of()
p2.be a part of()
Output:
Sq. of the number one is 1 | PID of the method 1125
Sq. of the quantity 2 is 4 | PID of the method 1125
Sq. of the quantity 3 is 9 | PID of the method 1125
Sq. of the quantity 4 is 16 | PID of the method 1125
Sq. of the quantity 5 is 25 | PID of the method 1126
Sq. of the quantity 6 is 36 | PID of the method 1126
Sq. of the quantity 7 is 49 | PID of the method 1126
Sq. of the quantity 8 is 64 | PID of the method 1126
We’ve noticed {that a} separate course of executes every record. Each have totally different course of IDs. To test whether or not our processes have been executed parallelly, we have to create a separate atmosphere, which we’ll focus on beneath.
Calculating Runtime With and With out Multiprocessing
To test whether or not we get a real parallelism, we’ll calculate the algorithm’s runtime with and with out multiprocessing.
For this, we would require an intensive record of integers that comprise greater than 10^6 integers. We will generate a listing utilizing random
library. We’ll use the time
module of Python to calculate the runtime. Under is the implementation for this. The code is self-explanatory, though you’ll be able to all the time have a look at the code feedback.
from multiprocessing import Course of
import os
import time
import random
def calculate_squares(numbers):
for num in numbers:
sq. = num * num
if __name__ == "__main__":
numbers = [
random.randrange(1, 50, 1) for i in range(10000000)
] # Making a random record of integers having measurement 10^7.
half = len(numbers) // 2
first_half = numbers[:half]
second_half = numbers[half:]
# ----------------- Creating Single Course of Atmosphere ------------------------#
start_time = time.time() # Begin time with out multiprocessing
p1 = Course of(
goal=calculate_squares, args=(numbers,)
) # Single course of P1 is executing all record
p1.begin()
p1.be a part of()
end_time = time.time() # Finish time with out multiprocessing
print(f"Execution Time With out Multiprocessing: {(end_time-start_time)*10**3}ms")
# ----------------- Creating Multi Course of Atmosphere ------------------------#
start_time = time.time() # Begin time with multiprocessing
p2 = Course of(goal=calculate_squares, args=(first_half,))
p3 = Course of(goal=calculate_squares, args=(second_half,))
p2.begin()
p3.begin()
p2.be a part of()
p3.be a part of()
end_time = time.time() # Finish time with multiprocessing
print(f"Execution Time With Multiprocessing: {(end_time-start_time)*10**3}ms")
Output:
Execution Time With out Multiprocessing: 619.8039054870605ms
Execution Time With Multiprocessing: 321.70287895202637ms
You possibly can observe that the time with multiprocessing is nearly half as in comparison with with out multiprocessing. This reveals that these two processes are executed concurrently at a time and present a habits of true parallelism.
You too can learn this text Sequential vs Concurrent vs Parallelism from Medium, which is able to assist you to to know the essential distinction between these Sequential, Concurrent and Parallel processes.
Aryan Garg is a B.Tech. Electrical Engineering scholar, presently within the last yr of his undergrad. His curiosity lies within the subject of Internet Growth and Machine Studying. He have pursued this curiosity and am desirous to work extra in these instructions.