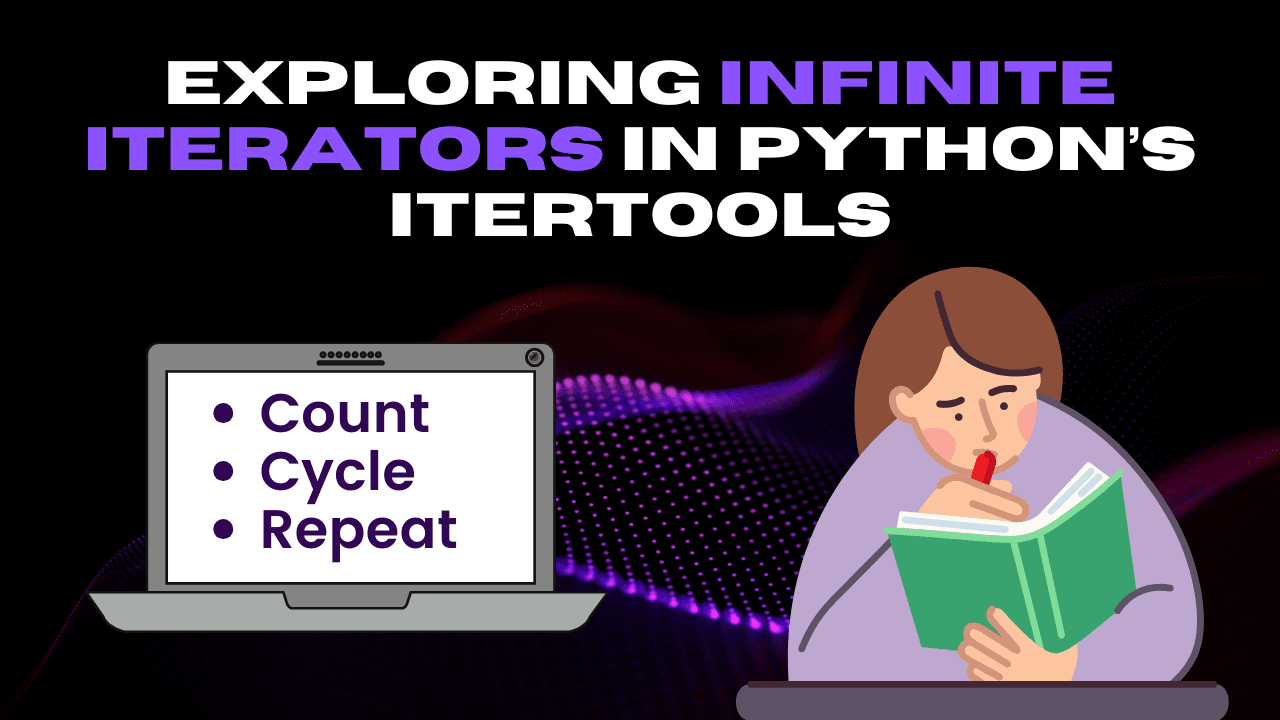
Picture by Writer
Infinite iterators, because the identify suggests, are particular varieties of iterators that may proceed producing values indefinitely. Not like the in-built iterators like lists, tuples, and dictionaries that ultimately attain an finish, infinite iterators can produce an never-ending stream of values. Such iterators are additionally typically known as infinite turbines or sequences. They discover use in numerous situations for fixing issues involving simulation, producing sequences, processing real-time knowledge, and extra.
The Itertools library in Python gives three in-built infinite iterators.
The rely() operate generates infinite numbers ranging from the desired worth and step measurement. The syntax for the rely iterator is as follows:
itertools.rely(begin=0, step=1)
It has two non-compulsory parameters: “begin” and “cease,” with default values of 0 and 1, respectively. “Begin” refers back to the preliminary worth of your counting, whereas “step” represents the increment used to advance the rely.
Allow us to analyze the operate with the assistance of an instance. If you want to generate a sequence of numbers with a step measurement of three similar to the desk of three, you should use this code:
from itertools import rely
counter = rely(3,3)
print("The desk of three is:")
for i in vary(10):
print(f"3 x {i+1} = {subsequent(counter)}")
Output
The desk of three is:
3 x 1 = 3
3 x 2 = 6
3 x 3 = 9
3 x 4 = 12
3 x 5 = 15
3 x 6 = 18
3 x 7 = 21
3 x 8 = 24
3 x 9 = 27
3 x 10 = 30
The cycle() operate creates an iterator and repeats all of the gadgets of the handed container indefinitely. Right here is the syntax for the cycle iterator:
itertools.cycle(iterable)
The “iterable” parameter right here could be any iterable knowledge construction in Python, akin to lists, tuples, units, and extra. Think about an instance of a site visitors gentle controller system that repeatedly cycles by completely different lights. No completely different actions are carried out whereas biking by the completely different coloured lights. We’ll use a wait time of 5 seconds to show our outcomes.
from itertools import cycle
import time
lights = ["red", "green", "yellow"]
cycle_iterator = cycle(lights)
whereas True:
print(f"Present gentle is: {subsequent(cycle_iterator)}")
time.sleep(5)
Output
Present gentle is: crimson
Present gentle is: inexperienced
Present gentle is: yellow
Present gentle is: crimson
Present gentle is: inexperienced
Present gentle is: yellow
You will note this output after roughly 25 seconds.
The repeat() operate generates a sequence of the desired quantity infinitely. It’s helpful when you want to generate a single worth indefinitely. The syntax for the repeat iterator is as follows:
itertools.repeat(worth, instances=inf)
We’ve two parameters right here: “worth” is for the quantity you need to generate infinitely, whereas “instances” is an non-compulsory parameter for what number of instances you need to generate that quantity. The default worth for “instances” is infinity, indicating that it’s going to print endlessly except you explicitly specify a finite quantity. For instance, if you want to generate the quantity “9” 3 times, then the next code can be utilized:
from itertools import repeat
iterator = repeat(9, 3)
for worth in iterator:
print(worth)
Output
These infinite iterators are extraordinarily useful in situations when we have to work with streams of information. The “rely”, “cycle” and “repeat” iterators present us the flexibility to unravel issues extra effectively and elegantly. Though utilizing them requires mandatory warning as they’ll result in infinite loops, when used thoughtfully they could be a invaluable useful resource for fixing programming issues. I hope you loved studying this text and when you’ve got something to share be at liberty to drop your strategies within the remark field under.
Kanwal Mehreen is an aspiring software program developer with a eager curiosity in knowledge science and purposes of AI in drugs. Kanwal was chosen because the Google Era Scholar 2022 for the APAC area. Kanwal likes to share technical information by writing articles on trending matters, and is obsessed with bettering the illustration of girls in tech business.
Kanwal Mehreen is an aspiring software program developer with a eager curiosity in knowledge science and purposes of AI in drugs. Kanwal was chosen because the Google Era Scholar 2022 for the APAC area. Kanwal likes to share technical information by writing articles on trending matters, and is obsessed with bettering the illustration of girls in tech business.